Section 2.4.3 : Python Dictionary Operations
Python dictionaries are incredibly powerful and versatile, making them a cornerstone of Python programming.
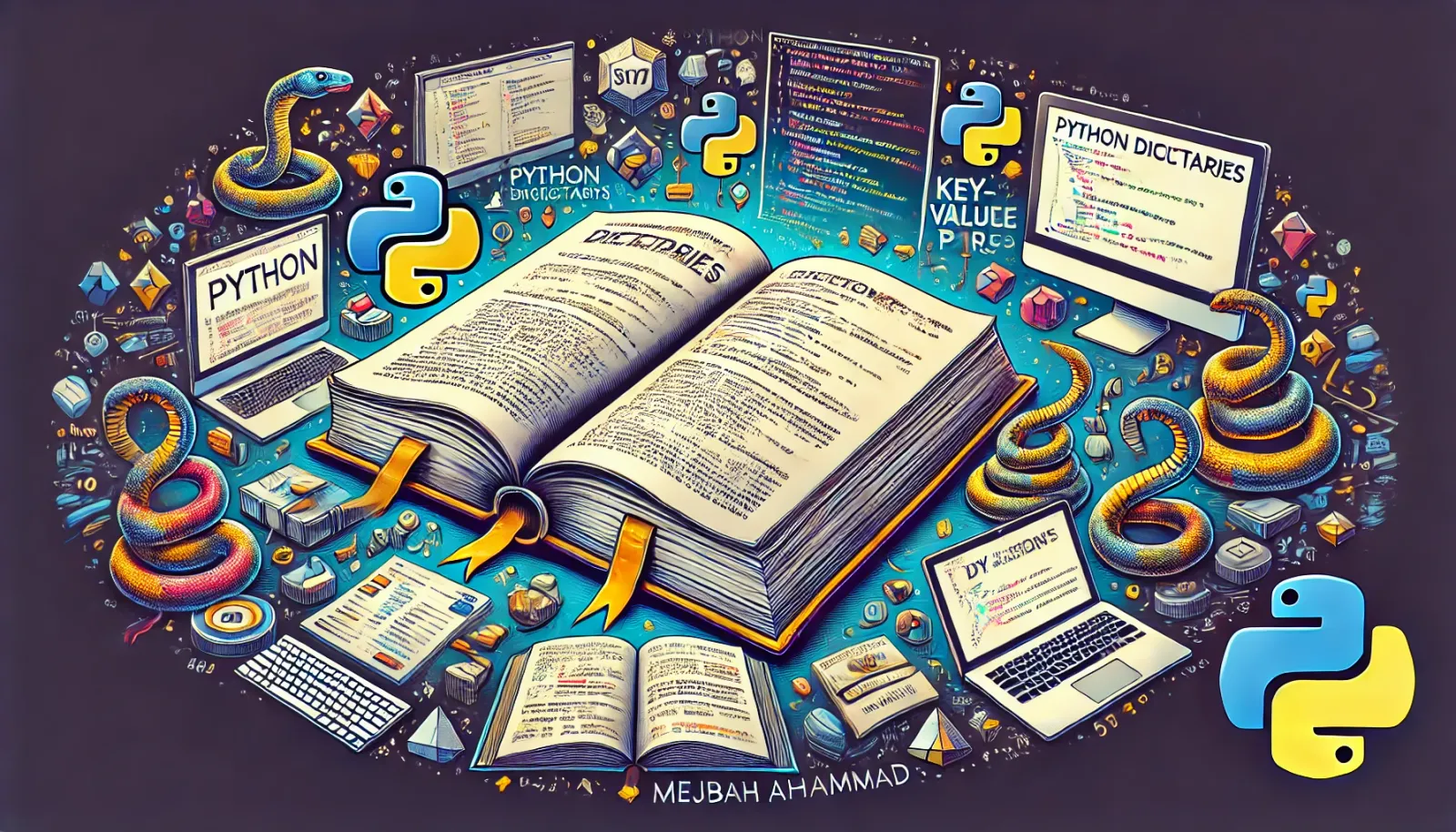
Python dictionaries are one of the most important and versatile data structures in Python. They allow you to store data in key-value pairs, which makes it easy to retrieve, update, and manage data efficiently. Dictionaries are widely used in various applications, from simple data storage to more complex operations like counting occurrences, grouping data, and implementing lookup tables.
In this guide, we will thoroughly explore Python dictionaries, covering their creation, manipulation, and methods with detailed explanations and coding examples. We will also discuss dictionary-specific operations, how dictionaries differ from other data structures, and their use cases. Each code example will be explained line by line to ensure a clear understanding of how dictionaries work in Python.
1. Creating Dictionaries
Dictionaries can be created in several ways:
- Using curly braces:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
Explanation:
- Line 1: A dictionary named
my_dict
is created with three key-value pairs:'name'
,'age'
, and'city'
. - Using the
dict()
constructor:
my_dict = dict(name='Alice', age=25, city='New York')
Explanation:
- Line 1: The
dict()
constructor creates a dictionarymy_dict
with the same key-value pairs as the previous example. - Creating an empty dictionary:
empty_dict = {}
Explanation:
- Line 1: An empty dictionary
empty_dict
is created using curly braces.
2. Accessing and Modifying Dictionary Elements
Dictionaries allow you to access and modify their elements using keys:
- Accessing values by key:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
name = my_dict['name']
print(name) # Output: Alice
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The value associated with the key
'name'
is accessed and stored in the variablename
. - Line 3: Printing
name
outputs"Alice"
. - Modifying values:
my_dict['age'] = 26
print(my_dict) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
Explanation:
- Line 1: The value associated with the key
'age'
is updated from25
to26
. - Line 2: Printing
my_dict
shows the updated dictionary. - Adding new key-value pairs:
my_dict['email'] = 'alice@example.com'
print(my_dict)
Explanation:
- Line 1: A new key-value pair
'email': 'alice@example.com'
is added to the dictionary. - Line 2: Printing
my_dict
shows the dictionary with the new entry. - Removing key-value pairs:
del my_dict['city']
print(my_dict) # Output: {'name': 'Alice', 'age': 26, 'email': 'alice@example.com'}
Explanation:
- Line 1: The key-value pair with the key
'city'
is removed from the dictionary using thedel
statement. - Line 2: Printing
my_dict
shows the dictionary after the deletion.
3. Dictionary Methods
Dictionaries come with a variety of built-in methods that make it easier to manipulate data.
get()
Purpose: Retrieves the value associated with a given key. If the key is not found, it returns a default value (which is None
by default).
Syntax:
value = dictionary.get(key, default_value)
Example:
my_dict = {'name': 'Alice', 'age': 25}
age = my_dict.get('age')
print(age) # Output: 25
# Trying to get a key that doesn't exist
email = my_dict.get('email', 'Not provided')
print(email) # Output: Not provided
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
get()
method retrieves the value associated with the key'age'
and stores it inage
. - Line 3: Printing
age
outputs25
. - Line 6: The
get()
method tries to retrieve the key'email'
. Since it doesn't exist, it returns the default value'Not provided'
. - Line 7: Printing
email
outputs'Not provided'
.
keys()
Purpose: Returns a view object that displays a list of all the keys in the dictionary.
Syntax:
keys_view = dictionary.keys()
Example:
my_dict = {'name': 'Alice', 'age': 25}
keys = my_dict.keys()
print(keys) # Output: dict_keys(['name', 'age'])
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
keys()
method returns a view object that contains the keys ofmy_dict
. - Line 3: Printing
keys
outputsdict_keys(['name', 'age'])
.
values()
Purpose: Returns a view object that displays a list of all the values in the dictionary.
Syntax:
values_view = dictionary.values()
Example:
my_dict = {'name': 'Alice', 'age': 25}
values = my_dict.values()
print(values) # Output: dict_values(['Alice', 25])
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
values()
method returns a view object that contains the values ofmy_dict
. - Line 3: Printing
values
outputsdict_values(['Alice', 25])
.
items()
Purpose: Returns a view object that displays a list of all the key-value pairs in the dictionary as tuples.
Syntax:
items_view = dictionary.items()
Example:
my_dict = {'name': 'Alice', 'age': 25}
items = my_dict.items()
print(items) # Output: dict_items([('name', 'Alice'), ('age', 25)])
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
items()
method returns a view object that contains the key-value pairs ofmy_dict
as tuples. - Line 3: Printing
items
outputsdict_items([('name', 'Alice'), ('age', 25)])
.
pop()
Purpose: Removes the specified key and returns the corresponding value. If the key is not found, a default value can be returned instead of raising an error.
Syntax:
value = dictionary.pop(key, default_value)
Example:
my_dict = {'name': 'Alice', 'age': 25}
age = my_dict.pop('age')
print(age) # Output: 25
print(my_dict) # Output: {'name': 'Alice'}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
pop()
method removes the key'age'
and returns its value, which is stored inage
. - Line 3: Printing
age
outputs25
. - Line 4: Printing
my_dict
shows the dictionary after the key'age'
has been removed.
popitem()
Purpose: Removes and returns the last inserted key-value pair as a tuple. Raises a KeyError
if the dictionary is empty.
Syntax:
key_value_pair = dictionary.popitem()
Example:
my_dict = {'name': 'Alice', 'age': 25}
last_item = my_dict.popitem()
print(last_item) # Output: ('age', 25)
print(my_dict) # Output: {'name': 'Alice'}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
popitem()
method removes the last inserted key-value pair and returns it as a
tuple, which is stored in last_item
.
- Line 3: Printing
last_item
outputs('age', 25)
. - Line 4: Printing
my_dict
shows the dictionary after the last inserted item has been removed.
update()
Purpose: Updates the dictionary with elements from another dictionary or an iterable of key-value pairs.
Syntax:
dictionary.update(other_dictionary_or_iterable)
Example:
my_dict = {'name': 'Alice', 'age': 25}
my_dict.update({'age': 26, 'city': 'New York'})
print(my_dict) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
update()
method updatesmy_dict
with new values for'age'
and a new key'city'
. - Line 3: Printing
my_dict
shows the updated dictionary.
clear()
Purpose: Removes all elements from the dictionary, leaving it empty.
Syntax:
dictionary.clear()
Example:
my_dict = {'name': 'Alice', 'age': 25}
my_dict.clear()
print(my_dict) # Output: {}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
clear()
method removes all elements frommy_dict
, leaving it empty. - Line 3: Printing
my_dict
outputs an empty dictionary{}
.
copy()
Purpose: Returns a shallow copy of the dictionary.
Syntax:
new_dict = dictionary.copy()
Example:
my_dict = {'name': 'Alice', 'age': 25}
copied_dict = my_dict.copy()
print(copied_dict) # Output: {'name': 'Alice', 'age': 25}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
copy()
method creates a shallow copy ofmy_dict
and stores it incopied_dict
. - Line 3: Printing
copied_dict
outputs{'name': 'Alice', 'age': 25}
.
fromkeys()
Purpose: Creates a new dictionary from a sequence of keys, with all values set to a specified value (or None
by default).
Syntax:
new_dict = dict.fromkeys(sequence_of_keys, value)
Example:
keys = ['name', 'age', 'city']
default_dict = dict.fromkeys(keys, 'unknown')
print(default_dict) # Output: {'name': 'unknown', 'age': 'unknown', 'city': 'unknown'}
Explanation:
- Line 1: A list
keys
is created with three elements. - Line 2: The
fromkeys()
method creates a dictionary with keys from thekeys
list, setting each value to'unknown'
. - Line 3: Printing
default_dict
outputs the new dictionary.
setdefault()
Purpose: Returns the value of a specified key. If the key does not exist, it inserts the key with a specified value (or None
by default) and returns that value.
Syntax:
value = dictionary.setdefault(key, default_value)
Example:
my_dict = {'name': 'Alice'}
age = my_dict.setdefault('age', 25)
print(age) # Output: 25
print(my_dict) # Output: {'name': 'Alice', 'age': 25}
Explanation:
- Line 1: A dictionary
my_dict
is created with one key-value pair. - Line 2: The
setdefault()
method checks if the key'age'
exists inmy_dict
. Since it doesn't, it adds the key with the value25
and returns25
. - Line 3: Printing
age
outputs25
. - Line 4: Printing
my_dict
shows the dictionary with the new key-value pair added.
4. Dictionary Comprehensions
Dictionary comprehensions provide a concise way to create dictionaries.
Example: Creating a Dictionary from a List
numbers = [1, 2, 3, 4, 5]
squares = {x: x**2 for x in numbers}
print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Explanation:
- Line 1: A list
numbers
is created with five elements. - Line 2: A dictionary comprehension is used to create a dictionary
squares
where each key is a number fromnumbers
and each value is the square of that number. - Line 3: Printing
squares
outputs the dictionary with numbers as keys and their squares as values.
Example: Filtering Dictionary Items
original_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
filtered_dict = {k: v for k, v in original_dict.items() if v % 2 == 0}
print(filtered_dict) # Output: {'b': 2, 'd': 4}
Explanation:
- Line 1: A dictionary
original_dict
is created. - Line 2: A dictionary comprehension is used to create
filtered_dict
, which only includes items fromoriginal_dict
where the value is even. - Line 3: Printing
filtered_dict
outputs{'b': 2, 'd': 4}
.
5. Iterating Over Dictionaries
You can iterate over a dictionary’s keys, values, or key-value pairs.
Example: Iterating Over Keys
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
for key in my_dict:
print(key)
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: A
for
loop is used to iterate over the keys inmy_dict
. - Line 3: Each key is printed. The output will be:
name
age
city
Example: Iterating Over Values
for value in my_dict.values():
print(value)
Explanation:
- Line 1: A
for
loop is used to iterate over the values inmy_dict
. - Line 2: Each value is printed. The output will be:
Alice
25
New York
Example: Iterating Over Key-Value Pairs
for key, value in my_dict.items():
print(f"{key}: {value}")
Explanation:
- Line 1: A
for
loop is used to iterate over the key-value pairs inmy_dict
using theitems()
method. - Line 2: Each key-value pair is printed. The output will be:
name: Alice
age: 25
city: New York
6. Checking for Key Existence
You can check if a key exists in a dictionary using the in
operator.
Example: Checking for a Key
my_dict = {'name': 'Alice', 'age': 25}
if 'age' in my_dict:
print("Key 'age' found")
else:
print("Key 'age' not found")
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: An
if
statement checks if the key'age'
exists inmy_dict
. - Line 3: If the key exists, a message is printed indicating that the key was found.
- Line 4: If the key does not exist, a message indicating that the key was not found would be printed (though this block is not reached in this example).
7. Nested Dictionaries
Dictionaries can contain other dictionaries as values, allowing for more complex data structures.
Example: Creating a Nested Dictionary
nested_dict = {
'person1': {'name': 'Alice', 'age': 25},
'person2': {'name': 'Bob', 'age': 30}
}
Explanation:
- Lines 1-3: A nested dictionary
nested_dict
is created, where each key ('person1'
and'person2'
) maps to another dictionary containing that person's name and age.
Accessing Nested Dictionary Elements
print(nested_dict['person1']['name']) # Output: Alice
Explanation:
- Line 1: The value associated with the key
'person1'
is another dictionary. The'name'
key of this nested dictionary is accessed to retrieve"Alice"
, which is then printed.
Modifying Nested Dictionary Elements
nested_dict['person1']['age'] = 26
print(nested_dict) # Output: {'person1': {'name': 'Alice', 'age': 26}, 'person2': {'name': 'Bob', 'age': 30}}
Explanation:
- Line 1: The
age
value for'person1'
is updated from25
to26
. - Line 2: Printing
nested_dict
shows the updated nested dictionary.
8. Merging Dictionaries
Dictionaries can be merged using the update()
method or the |
operator (Python 3.9+).
Example: Merging with update()
dict1 = {'name': 'Alice', 'age': 25}
dict2 = {'age': 26, 'city': 'New York'}
dict1.update(dict2)
print(dict1) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
Explanation:
- Line 1: A dictionary
dict1
is created. - Line 2: A dictionary
dict2
is created with an overlapping key'age'
and a new key'city'
. - Line 3: The
update()
method mergesdict2
intodict1
, updating the'age'
and adding the'city'
. - Line 4: Printing
dict1
shows the merged dictionary.
Example: Merging with |
Operator
dict3 = dict1 | dict2
print(dict3) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
Explanation:
- Line 1: The
|
operator is used to mergedict1
anddict2
into a new dictionarydict3
. - Line 2: Printing
dict3
shows the merged dictionary.
9. Using Dictionaries as Caches
Dictionaries are often used as caches to store expensive or frequently accessed computations.
Example: Implementing a Simple Cache
cache = {}
def fib(n):
if n in cache:
return cache[n]
if n <= 1:
return n
result = fib(n-1) + fib(n-2)
cache[n] = result
return result
print(fib(10)) # Output: 55
print(cache) # Output: {2: 1, 3: 2, 4: 3, 5: 5, 6: 8, 7: 13, 8: 21, 9: 34, 10: 55}
Explanation:
- Line 1: An empty dictionary
cache
is created. - Line 3: The
fib()
function is defined to calculate the nth Fibonacci number. - Line 4: If
n
is in the cache, the cached value is returned. - Line 5: The base case of the Fibonacci sequence is handled.
- Line 8: The computed Fibonacci number is stored in the cache.
- Line 10: The Fibonacci number for
n=10
is calculated and printed, which is55
. - Line 11: Printing
cache
shows the cached Fibonacci numbers, speeding up subsequent calculations.
10. Counting and Grouping with Dictionaries
Dictionaries are ideal for counting occurrences or grouping data.
Example: Counting Occurrences of Elements
words = ['apple', 'banana', 'apple', 'orange', 'banana', 'banana']
word_count = {}
for word in words:
word_count[word] = word_count.get(word, 0) + 1
print(word_count) # Output: {'apple': 2, 'banana': 3, 'orange': 1}
Explanation:
- Line 1: A list
words
is created with repeated elements. - Line 2: An empty dictionary
word_count
is created to store the counts. - Line 3: A
for
loop iterates over each word inwords
. - Line 4: The
get()
method retrieves the current count of the word (defaulting to0
if not found) and increments it by1
. - Line 6: Printing
word_count
outputs the dictionary with the count of each word.
Example: Grouping Elements by Key
students = [
{'name': 'Alice', 'grade': 'A'},
{'name': 'Bob', 'grade': 'B'},
{'name': 'Charlie', 'grade': 'A'},
{'name': 'David', 'grade': 'C'}
]
grouped_by_grade = {}
for student in students:
grade = student['grade']
if grade not in grouped_by_grade:
grouped_by_grade[grade] = []
grouped_by_grade[grade].append(student['name'])
print(grouped_by_grade) # Output: {'A': ['Alice', 'Charlie'], 'B': ['Bob'], 'C': ['David']}
Explanation:
- Line 1: A list of dictionaries
students
is created, where each dictionary contains a student's name and grade. - Line 6: An empty dictionary
grouped_by_grade
is created to group students by their grades. - Lines 7-10: A
for
loop iterates over thestudents
, grouping names by theirgrade
. - Line 11: Printing
grouped_by_grade
shows the dictionary where each key is a grade and each value is a list of students with that grade.
11. Sorting Dictionaries
Dictionaries can be sorted by keys, values, or custom criteria.
Example: Sorting by Keys
my_dict = {'b': 3, 'a': 2, 'c': 1}
sorted_by_key = dict(sorted(my_dict.items()))
print(sorted_by_key) # Output: {'a': 2, 'b': 3, 'c': 1}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
sorted()
function sorts the items by key, and thedict()
constructor creates a new sorted dictionarysorted_by_key
. - Line 3: Printing
sorted_by_key
shows the dictionary sorted by keys.
Example: Sorting by Values
sorted_by_value = dict(sorted(my_dict.items(), key=lambda item: item[1]))
print(sorted_by_value) # Output: {'c': 1, 'a': 2, 'b': 3}
Explanation:
- Line 1: The
sorted()
function sorts the items by value using a lambda function as the key. - Line 2: Printing
sorted_by_value
shows the dictionary sorted by values.
12. Using Dictionaries with Functions
Dictionaries can be passed to functions, returned from functions, and used as function arguments.
Example: Passing a Dictionary to a Function
def print_person_info(person):
for key, value in person.items():
print(f"{key}: {value}")
person_info = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print_person_info(person_info)
Explanation:
- Line 1: The
print_person_info()
function is defined to print key-value pairs in a dictionary. - Line 5: A dictionary
person_info
is created. - Line 6: The
print_person_info()
function is called withperson_info
as the argument.
Example: Returning a Dictionary from a Function
def create_person(name, age, city):
return {'name': name, 'age': age, 'city': city}
person = create_person('Alice', 25, 'New York')
print(person) # Output: {'name': 'Alice', 'age': 25, 'city': 'New York'}
Explanation:
- Line 1: The
create_person()
function is defined to create and return a dictionary with the given name, age, and city. - Line 5: The function is called to create a dictionary
person
. - Line 6: Printing
person
outputs the created dictionary.
Example: Using a Dictionary to Pass Keyword Arguments
def describe_city(city, country, population):
print(f"{city} is in {country} with a population of {population}.")
city_info = {'city': 'New York', 'country': 'USA', 'population': 8419000}
describe_city(**city_info)
Explanation:
- Line 1: The
describe_city()
function is defined to print information about a city. - Line 5: A dictionary
city_info
is created with keys corresponding to the function's parameters. - Line 6: The
**city_info
syntax unpacks the dictionary into keyword arguments, passing them to the function.
13. Advanced Dictionary Techniques
In addition to the basic operations and methods, Python dictionaries can be used in advanced scenarios, such as handling more complex data structures, optimizing performance, and integrating with other Python features.
13.1. Handling Missing Keys with defaultdict
Python's collections
module provides a defaultdict
class, which is a subclass of dict
. It is useful when dealing with missing keys because it allows you to define a default value or a function that generates a default value automatically when accessing a non-existent key.
Example: Using defaultdict
for Counting
from collections import defaultdict
word_list = ['apple', 'banana', 'apple', 'orange', 'banana', 'banana']
word_count = defaultdict(int)
for word in word_list:
word_count[word] += 1
print(word_count) # Output: defaultdict(<class 'int'>, {'apple': 2, 'banana': 3, 'orange': 1})
Explanation:
- Line 1: The
defaultdict
class is imported from thecollections
module. - Line 3: A list
word_list
is created with repeated words. - Line 4: A
defaultdict
namedword_count
is created, withint
as the default factory function. This means that any non-existent key accessed will automatically have a default value of0
. - Lines 6-7: A
for
loop iterates over each word inword_list
, incrementing the count for each word inword_count
. - Line 9: Printing
word_count
shows the count of each word as adefaultdict
object.
13.2. Nested Dictionaries with defaultdict
When working with nested dictionaries, using a defaultdict
can simplify the process of adding elements to the nested levels.
Example: Creating Nested Dictionaries
nested_dict = defaultdict(lambda: defaultdict(int))
nested_dict['A']['x'] += 1
nested_dict['A']['y'] += 2
nested_dict['B']['x'] += 3
print(nested_dict)
# Output: defaultdict(<function <lambda> at 0x...>, {'A': defaultdict(<class 'int'>, {'x': 1, 'y': 2}), 'B': defaultdict(<class 'int'>, {'x': 3})})
Explanation:
- Line 1: A
defaultdict
namednested_dict
is created, where each key at the first level is associated with anotherdefaultdict
withint
as the default factory. - Lines 3-5: Elements are added to the nested dictionaries by incrementing values. The
defaultdict
ensures that the nested dictionaries are created automatically if they don't exist. - Line 7: Printing
nested_dict
shows the nested structure with incremented values.
13.3. Combining Dictionaries Using ChainMap
The ChainMap
class from the collections
module allows you to combine multiple dictionaries into a single view. This can be particularly useful when you want to treat multiple dictionaries as a single mapping.
Example: Combining Dictionaries with ChainMap
from collections import ChainMap
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
combined = ChainMap(dict1, dict2)
print(combined['a']) # Output: 1
print(combined['b']) # Output: 2
print(combined['c']) # Output: 4
Explanation:
- Line 1: The
ChainMap
class is imported from thecollections
module. - Lines 3-4: Two dictionaries
dict1
anddict2
are created. - Line 5: A
ChainMap
object namedcombined
is created, which combinesdict1
anddict2
. If a key appears in multiple dictionaries, the value from the first dictionary in the chain is used. - Lines 7-9: The values associated with keys
'a'
,'b'
, and'c'
are accessed fromcombined
. The value for'b'
comes fromdict1
because it appears first in the chain.
13.4. Using Counter
for Counting Elements
The Counter
class from the collections
module is a specialized dictionary designed for counting hashable objects. It's useful for counting occurrences in a dataset.
Example: Counting Elements with Counter
from collections import Counter
word_list = ['apple', 'banana', 'apple', 'orange', 'banana', 'banana']
word_count = Counter(word_list)
print(word_count) # Output: Counter({'banana': 3, 'apple': 2, 'orange': 1})
Explanation:
- Line 1: The
Counter
class is imported from thecollections
module. - Line 3: A list
word_list
is created with repeated words. - Line 4: A
Counter
object namedword_count
is created by passingword_list
to theCounter
constructor. - Line 6: Printing
word_count
shows the count of each word, similar to a dictionary but with additional methods for counting.
13.5. Dictionary Views and Dynamic Updates
Dictionary view objects (returned by keys()
, values()
, and items()
) are dynamic and reflect any changes made to the dictionary.
Example: Dynamic Updates in Dictionary Views
my_dict = {'a': 1, 'b': 2}
keys_view = my_dict.keys()
values_view = my_dict.values()
print(keys_view) # Output: dict_keys(['a', 'b'])
print(values_view) # Output: dict_values([1, 2])
my_dict['c'] = 3
print(keys_view) # Output: dict_keys(['a', 'b', 'c'])
print(values_view) # Output: dict_values([1, 2, 3])
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: The
keys_view
object is created using thekeys()
method. - Line 3: The
values_view
object is created using thevalues()
method. - Lines 5-6: The initial keys and values in the dictionary are printed.
- Line 8: A new key-value pair
'c': 3
is added tomy_dict
. - Lines 10-11: The updated keys and values are printed, showing that the view objects reflect the changes dynamically.
13.6. Using Dictionaries for Lookup Tables
Dictionaries are ideal for implementing lookup tables, which map input values to corresponding outputs.
Example: Simple Lookup Table
lookup_table = {
'a': 1,
'b': 2,
'c': 3
}
letter = 'b'
number = lookup_table.get(letter, 'Not found')
print(f"The number for '{letter}' is {number}.") # Output: The number for 'b' is 2.
Explanation:
- Line 1: A dictionary
lookup_table
is created with letters as keys and corresponding numbers as values. - Line 6: The letter
'b'
is looked up inlookup_table
using theget()
method. If the key is not found, it returns'Not found'
. - Line 7: The result is printed, showing that the number for
'b'
is2
.
13.7. Optimizing Performance with OrderedDict
In Python versions before 3.7, dictionaries did not maintain the order of keys. The OrderedDict
from the collections
module was used to preserve key order. From Python 3.7 onwards, dictionaries maintain insertion order by default, but OrderedDict
still provides additional features like reordering elements.
Example: Using OrderedDict
from collections import OrderedDict
ordered_dict = OrderedDict([('a', 1), ('b', 2), ('c', 3)])
print(ordered_dict) # Output: OrderedDict([('a', 1), ('b', 2), ('c', 3)])
ordered_dict.move_to_end('b')
print(ordered_dict) # Output: OrderedDict([('a', 1), ('c', 3), ('b', 2)])
Explanation:
- Line 1: The
OrderedDict
class is imported from thecollections
module. - Line 3: An
OrderedDict
namedordered_dict
is created with three key-value pairs in a specific order. - Line 5: The
move_to_end()
method is used to move the key'b'
to the end of the dictionary. - Line 6: Printing
ordered_dict
shows the updated order of the dictionary elements.
13.8. Inverting Dictionaries
Inverting a dictionary means swapping its keys and values. This is often useful when you need to perform a reverse lookup.
Example: Inverting a Dictionary
my
_dict = {'a': 1, 'b': 2, 'c': 3}
inverted_dict = {v: k for k, v in my_dict.items()}
print(inverted_dict) # Output: {1: 'a', 2: 'b', 3: 'c'}
Explanation:
- Line 1: A dictionary
my_dict
is created. - Line 2: A dictionary comprehension is used to invert
my_dict
, swapping its keys and values, and storing the result ininverted_dict
. - Line 3: Printing
inverted_dict
shows the inverted dictionary.
14. Common Use Cases for Dictionaries
Dictionaries are frequently used in various real-world applications due to their flexibility and efficiency in managing key-value data.
14.1. Storing Configuration Settings
Dictionaries are commonly used to store configuration settings for applications because they allow easy access and modification of settings by key.
Example: Application Configuration
config = {
'host': 'localhost',
'port': 8080,
'debug': True
}
print(f"Host: {config['host']}, Port: {config['port']}, Debug: {config['debug']}")
Explanation:
- Line 1: A dictionary
config
is created to store application configuration settings such as host, port, and debug mode. - Line 5: The configuration settings are accessed by key and printed.
14.2. Counting Occurrences in Large Datasets
Dictionaries are often used to count occurrences of elements in large datasets, such as words in a document or items in a transaction log.
Example: Counting Word Frequencies
import re
from collections import Counter
text = "apple banana apple orange banana banana"
words = re.findall(r'\w+', text.lower())
word_count = Counter(words)
print(word_count) # Output: Counter({'banana': 3, 'apple': 2, 'orange': 1})
Explanation:
- Line 1: The
re
andCounter
modules are imported. - Line 3: A string
text
is created containing repeated words. - Line 4: The
findall()
function from there
module is used to extract all words from the text. - Line 5: A
Counter
object namedword_count
is created to count the frequency of each word. - Line 7: Printing
word_count
shows the count of each word.
14.3. Implementing Switch Cases (Emulating Switch Statements)
Python does not have a built-in switch
statement like other languages. However, you can emulate this functionality using dictionaries.
Example: Emulating a Switch Statement
def switch_case(value):
switch = {
1: "Case 1",
2: "Case 2",
3: "Case 3"
}
return switch.get(value, "Default Case")
print(switch_case(1)) # Output: Case 1
print(switch_case(4)) # Output: Default Case
Explanation:
- Line 1: The
switch_case()
function is defined, which emulates a switch statement using a dictionary. - Line 2: A dictionary
switch
is created with cases as keys and corresponding output strings as values. - Line 6: The
get()
method retrieves the value associated with the given key (value
). If the key is not found, it returns"Default Case"
. - Line 8: The function is tested with
1
, which returns"Case 1"
. - Line 9: The function is tested with
4
, which returns the default case"Default Case"
.
14.4. Caching Computed Results
Dictionaries are frequently used to cache the results of expensive computations to avoid redundant processing.
Example: Memoization with a Dictionary
cache = {}
def expensive_computation(n):
if n in cache:
return cache[n]
result = n ** 2 # Simulate an expensive operation
cache[n] = result
return result
print(expensive_computation(10)) # Output: 100
print(expensive_computation(10)) # Output: 100 (retrieved from cache)
Explanation:
- Line 1: A dictionary
cache
is created to store the results of computations. - Line 3: The
expensive_computation()
function is defined, which checks if the result forn
is in the cache. - Lines 4-5: If
n
is found in the cache, the cached result is returned. - Line 7: If
n
is not in the cache, the computation is performed (simulated here asn ** 2
), and the result is stored in the cache. - Line 8: The result is returned.
- Lines 10-11: The function is tested with
10
. The first call computes and caches the result, while the second call retrieves the result from the cache.
15. Performance Considerations with Dictionaries
When working with large dictionaries or performance-critical applications, it's important to consider the efficiency of dictionary operations.
15.1. Dictionary Performance
Dictionaries in Python are implemented using hash tables, which generally provide O(1) time complexity for lookups, insertions, and deletions. This makes them highly efficient for scenarios where fast access to data is required.
Example: Measuring Performance
import time
large_dict = {i: i**2 for i in range(1000000)}
start_time = time.time()
value = large_dict[999999]
end_time = time.time()
print(f"Lookup time: {end_time - start_time} seconds")
Explanation:
- Line 1: The
time
module is imported to measure performance. - Line 3: A large dictionary
large_dict
is created with one million key-value pairs. - Line 5: The current time is recorded in
start_time
. - Line 6: A lookup operation is performed to access the value associated with the key
999999
. - Line 7: The time after the lookup is recorded in
end_time
. - Line 9: The time taken for the lookup operation is printed, typically showing a very small value due to the O(1) time complexity.
15.2. Memory Efficiency
While dictionaries are efficient in terms of time complexity, they may consume more memory than other data structures like lists, especially when storing large numbers of key-value pairs. This is because dictionaries allocate extra space to maintain their hash tables and reduce the likelihood of collisions.
Example: Measuring Memory Usage
import sys
small_list = [i for i in range(1000)]
small_dict = {i: i for i in range(1000)}
print(f"List size: {sys.getsizeof(small_list)} bytes")
print(f"Dictionary size: {sys.getsizeof(small_dict)} bytes")
Explanation:
- Line 1: The
sys
module is imported to measure memory usage. - Lines 3-4: A list
small_list
and a dictionarysmall_dict
are created with 1000 elements. - Lines 6-7: The memory usage of the list and the dictionary is printed using the
getsizeof()
function. The dictionary typically uses more memory due to its underlying structure.
15.3. Reducing Memory Usage with __slots__
In scenarios where memory usage is a concern, you can use the __slots__
attribute in custom classes to reduce the memory footprint. While this is not directly related to dictionaries, it’s useful when creating objects stored in dictionaries.
Example: Using __slots__
in a Custom Class
class Person:
__slots__ = ['name', 'age']
def __init__(self, name, age):
self.name = name
self.age = age
p = Person('Alice', 30)
print(p.name, p.age) # Output: Alice 30
Explanation:
- Line 1: The
Person
class is defined with__slots__
to restrict the attributes it can hold. - Lines 2-3: The
__slots__
attribute specifies thatPerson
objects will only havename
andage
attributes, which reduces memory usage. - Lines 5-6: The
__init__()
method initializes thename
andage
attributes. - Lines 8-9: An instance of
Person
is created and its attributes are accessed and printed.
Conclusion
Python dictionaries are a highly flexible and powerful data structure that allows you to efficiently store and manipulate data using key-value pairs. Throughout this guide, we explored the creation, manipulation, and various methods available for working with dictionaries. From simple operations like adding and removing elements to more complex tasks like sorting, merging, and using dictionaries in functions, you now have a comprehensive understanding of how to leverage dictionaries in Python programming.
Dictionaries are essential for many programming tasks, such as data storage, counting, grouping, and implementing caches. Their versatility and performance make them a fundamental tool in a Python developer's toolkit, enabling the efficient handling of structured data in a wide range of applications.