Section 2.3: Text Type
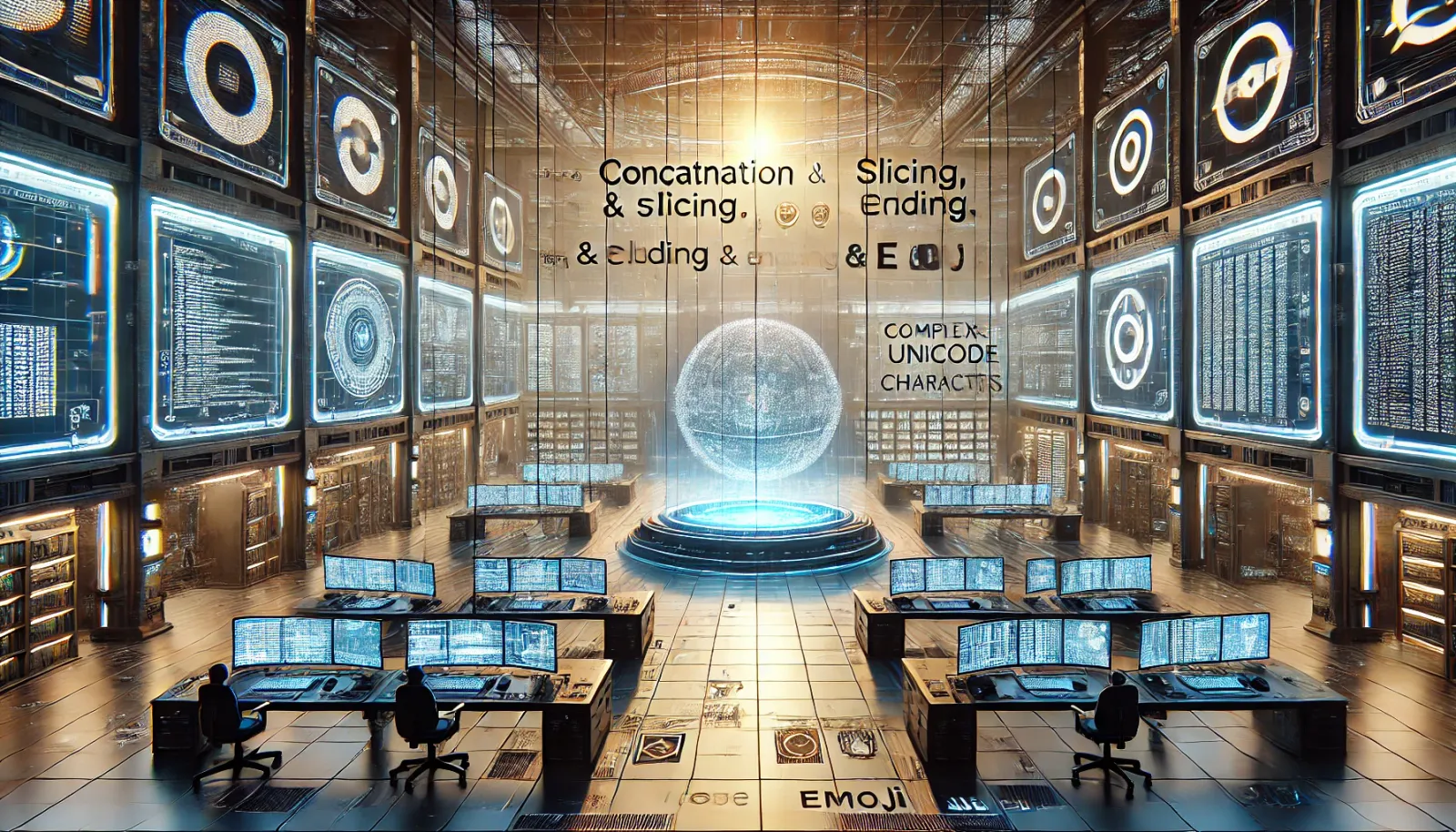
✏️ Basics of Strings: Creation and Basic Operations
Strings are one of the most commonly used data types in Python, representing sequences of characters. In Python, strings are immutable, meaning that once a string is created, it cannot be modified. Understanding how to create, manipulate, and perform operations on strings is fundamental to working with text data.
Creating Strings
In Python, strings can be created using single quotes ('
), double quotes ("
), or triple quotes ('''
or """
). Triple quotes are particularly useful for creating multi-line strings or strings that contain both single and double quotes.
# Creating strings in Python
single_quoted = 'Hello, World!' # Using single quotes
double_quoted = "Hello, World!" # Using double quotes
multi_line = '''This is a
multi-line string''' # Using triple quotes for multi-line string
Explanation:
- Line 2:
single_quoted
is a string created using single quotes. - Line 3:
double_quoted
is a string created using double quotes. Both are equivalent for single-line strings. - Line 4:
multi_line
is a multi-line string created using triple quotes, allowing text to span multiple lines.
Basic String Operations
Strings in Python support various operations, such as concatenation, repetition, and slicing.
# Basic string operations
greeting = "Hello"
name = "Alice"
# Concatenation
full_greeting = greeting + ", " + name + "!"
print(full_greeting) # Output: Hello, Alice!
# Repetition
echo = greeting * 3
print(echo) # Output: HelloHelloHello
# Slicing
first_letter = name[0] # Accessing the first character
substring = name[1:4] # Accessing a substring
Explanation:
- Line 3:
full_greeting
concatenatesgreeting
, a comma,name
, and an exclamation mark. - Line 7:
echo
repeats the stringgreeting
three times. - Line 10:
first_letter
retrieves the first character ofname
. - Line 11:
substring
retrieves a portion of the string from index 1 to 3.
String Length and Membership
Python provides built-in functions to determine the length of a string and to check for the presence of a substring within a string.
# Length and membership
message = "Python programming"
# Length of the string
length = len(message)
print(length) # Output: 18
# Membership check
contains_python = "Python" in message
print(contains_python) # Output: True
Explanation:
- Line 4:
len(message)
returns the number of characters inmessage
. - Line 7:
"Python" in message
checks if the substring "Python" is present inmessage
, returningTrue
.
🔧 Advanced String Manipulation: Methods and Formatting
Python provides a rich set of methods for advanced string manipulation, including methods for searching, replacing, formatting, and transforming strings. These methods make it easier to work with text data in various contexts.
String Methods for Searching and Replacing
Python strings come with several built-in methods to search for and replace substrings.
# Searching and replacing in strings
text = "The quick brown fox jumps over the lazy dog"
# Finding a substring
position = text.find("fox")
print(position) # Output: 16
# Replacing a substring
new_text = text.replace("fox", "cat")
print(new_text) # Output: The quick brown cat jumps over the lazy dog
Explanation:
- Line 4:
text.find("fox")
searches for the substring "fox" and returns the starting index (16
). - Line 7:
text.replace("fox", "cat")
replaces "fox" with "cat", creating a new string.
String Transformation Methods
Python provides methods to change the case of strings, strip unwanted characters, and split or join strings.
# String transformation methods
original = " Python Programming "
# Changing case
lowercase = original.lower()
uppercase = original.upper()
# Stripping whitespace
stripped = original.strip()
# Splitting and joining
words = original.split() # Splits into a list of words
joined = "-".join(words) # Joins with a hyphen
print(lowercase) # Output: python programming
print(uppercase) # Output: PYTHON PROGRAMMING
print(stripped) # Output: Python Programming
print(words) # Output: ['Python', 'Programming']
print(joined) # Output: Python-Programming
Explanation:
- Line 5:
lowercase
converts the string to lowercase. - Line 6:
uppercase
converts the string to uppercase. - Line 9:
stripped
removes leading and trailing whitespace. - Line 12:
split()
breaks the string into a list of words based on whitespace. - Line 13:
join()
combines the words into a single string with hyphens as separators.
String Formatting
Python offers several ways to format strings, including using the format()
method and f-strings (available in Python 3.6 and later).
# String formatting
name = "Alice"
age = 30
# Using format() method
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string) # Output: My name is Alice and I am 30 years old.
# Using f-strings
formatted_fstring = f"My name is {name} and I am {age} years old."
print(formatted_fstring) # Output: My name is Alice and I am 30 years old.
Explanation:
- Line 6:
format()
insertsname
andage
into the string in the specified order. - Line 10: The f-string
{name}
and{age}
embed variables directly within the string, providing a more concise and readable format.
String Escaping and Raw Strings
Sometimes, strings contain characters that need to be escaped, such as quotes or backslashes. Python allows you to escape these characters using a backslash (\
). Alternatively, you can use raw strings, which treat backslashes as literal characters.
# String escaping and raw strings
escaped = "She said, \"Hello!\""
raw_string = r"C:\Users\Alice\Documents"
print(escaped) # Output: She said, "Hello!"
print(raw_string) # Output: C:\Users\Alice\Documents
Explanation:
- Line 2: The backslash in
escaped
allows the double quotes to be included in the string. - Line 3: The
r
prefix before the string denotes a raw string, which treats backslashes as literal characters rather than escape sequences.
🌍 Unicode in Python: Handling Non-ASCII Text
Unicode is a standard for representing a vast range of characters from different languages and scripts. Python’s native string type (in Python 3) is Unicode, making it easy to work with non-ASCII text. This is particularly important in a globalized world where applications often need to handle diverse languages and symbols.
Basics of Unicode in Python
In Python 3, strings are Unicode by default. This means you can include characters from any language or script in your strings without special handling.
# Unicode strings in Python
japanese = "こんにちは" # Japanese for "Hello"
emoji = "😊" # Emoji as a string
print(japanese) # Output: こんにちは
print(emoji) # Output: 😊
Explanation:
- Line 2:
japanese
is a string containing Japanese characters. - Line 3:
emoji
contains an emoji, demonstrating that Python strings can include any Unicode character.
Encoding and Decoding Strings
While Python strings are Unicode by default, you may need to encode or decode strings when working with files, networks, or APIs that require specific encodings like UTF-8.
# Encoding and decoding strings
text = "Café"
# Encoding the string to bytes
encoded_text = text.encode('utf-8')
print(encoded_text) # Output: b'Caf\xc3\xa9'
# Decoding bytes back to a string
decoded_text = encoded_text.decode('utf-8')
print(decoded_text) # Output: Café
Explanation:
- Line 5:
encode('utf-8')
converts the stringtext
into a sequence of bytes using the UTF-8 encoding. - Line 9:
decode('utf-8')
converts the byte sequence back into a string.
Handling Special Characters and Normalization
When dealing with text from different sources, special characters might be represented differently. Unicode normalization can be used to standardize these representations.
import unicodedata
# Unicode normalization
s1 = "Café"
s2 = "Cafe\u0301" # 'é' as 'e' + combining accent
# Normalizing to NFC form
normalized_s1 = unicodedata.normalize('NFC', s1)
normalized_s2 = unicodedata.normalize('NFC', s2)
# Checking if the strings are equivalent after normalization
are_equal = normalized_s1 == normalized_s2
print(are_equal) # Output: True
Explanation:
- Line 8:
normalize('NFC', s1)
converts the strings1
into its canonical form. - Line 9: Similarly,
s2
is normalized to its canonical form. - Line 12:
are_equal
checks if the normalized versions ofs1
ands2
are equivalent, which they are, despite their initial differences.
Working with Non-English Languages
Python’s Unicode support makes it straightforward to work with text in non-English languages. This is especially useful in applications that need to handle internationalization (i18n) or localization (l10n).
# Working with non-English languages
chinese_text = "你好" # Chinese for "Hello"
arabic_text = "مرحبا" # Arabic for "Hello"
russian_text = "Привет" # Russian for "Hello"
print(chinese_text) # Output: 你好
print(arabic_text) # Output: مرحبا
print(russian_text) # Output: Привет
Explanation:
- Lines 2-4: Strings
chinese_text
,arabic_text
, andrussian_text
contain greetings in Chinese, Arabic, and Russian, respectively. Python handles these scripts natively without any additional encoding or decoding.
Practical Example: Reading and Writing Files with Unicode
Handling Unicode properly is critical when reading from or writing to text files that may contain non-ASCII characters.
# Reading and writing files with Unicode
filename = "example.txt"
# Writing Unicode text to a file
with open(filename, 'w', encoding='utf-8') as file:
file.write("Café\n")
file.write("こんにちは\n")
file.write("مرحبا\n")
# Reading Unicode text from a file
with open(filename, 'r', encoding='utf-8') as file:
content = file.read()
print(content)
Explanation:
- Line 6: The file
example.txt
is opened in write mode ('w'
), and the encoding is explicitly set to UTF-8 to handle Unicode characters. - Lines 7-9: Unicode text, including accented characters and non-Latin scripts, is written to the file.
- Line 13: The file is reopened in read mode (
'r'
) with UTF-8 encoding to correctly read the Unicode text. - Line 14: The content of the file is read and printed, showing that Python handles the text correctly.
🛠️ Best Practices for Working with Strings
Working with strings in Python involves several best practices to ensure your code is efficient, readable, and robust when handling text data, especially when dealing with Unicode.
Normalize Unicode Text: To avoid issues with different representations of the same characters, normalize Unicode strings before processing or comparison.
normalized_text = unicodedata.normalize('NFC', text)
Handle Encodings Explicitly: Always specify the encoding when reading from or writing to files, especially when dealing with non-ASCII text.
with open("file.txt", "r", encoding="utf-8") as file:
content = file.read()
Be Cautious with Mutable Sequences: Since strings are immutable, operations that modify a string (e.g., concatenation in a loop) can lead to inefficient code. Consider using lists or str.join()
for such operations.
# Inefficient string concatenation in a loop
result = ""
for word in ["Hello", "World"]:
result += word + " "
# More efficient approach using join
result = " ".join(["Hello", "World"])
Use Raw Strings for Regular Expressions: When working with regular expressions or paths, use raw strings to avoid issues with backslashes.
regex_pattern = r"\d{3}-\d{2}-\d{4}" # A regular expression pattern
🔗 Resources for Further Reading
- Python Official Documentation on Strings
- This section of the Python documentation covers the
str
type in detail, including methods, formatting, and Unicode handling.
- This section of the Python documentation covers the
- Real Python: Python Strings
- An in-depth article that explores Python strings, covering everything from basic operations to advanced manipulation and formatting techniques.
- W3Schools: Python Strings
- A beginner-friendly guide that introduces Python strings, along with practical examples and explanations of common string methods.
- Geeks for Geeks: Python String Methods
- A comprehensive list of Python string methods with examples, including explanations of how and when to use each method.
- The Unicode Consortium: Unicode Standard
- The official source for understanding Unicode, its structure, and its importance in text processing and internationalization.
- Python's
unicodedata
Module Documentation- Documentation for the
unicodedata
module, which provides access to the Unicode character database, allowing for advanced text processing tasks.
- Documentation for the
- Stack Overflow: Handling Unicode Strings in Python
- A discussion on Stack Overflow that addresses common issues and best practices for working with Unicode strings in Python.
- Python's
re
Module Documentation- Official documentation for the
re
module, covering regular expressions in Python, often used in conjunction with strings.
- Official documentation for the
- Automate the Boring Stuff with Python: Working with Strings
- A chapter from the popular book "Automate the Boring Stuff with Python" that focuses on string manipulation, providing practical examples for common tasks.
- Learn Python the Hard Way: Strings
- An exercise from "Learn Python the Hard Way" that introduces strings and basic operations, suitable for beginners looking to understand the fundamentals.
In conclusion, Python's string type is a versatile and powerful tool for handling text data. From basic creation and manipulation to advanced formatting and Unicode handling, mastering strings in Python is essential for any programmer. By following best practices and utilizing the wide range of built-in methods and functions, you can efficiently manage and process text in your applications. The resources provided will further enhance your understanding and ability to work with strings in Python.