🗃️ Section 1.5: Collections in Python
Lists, Tuples, Sets, and Dictionary Overview Lists: A list is an ordered and mutable collection used to store a series of items. Lists are created by placing elements inside square brackets [], separated by commas.
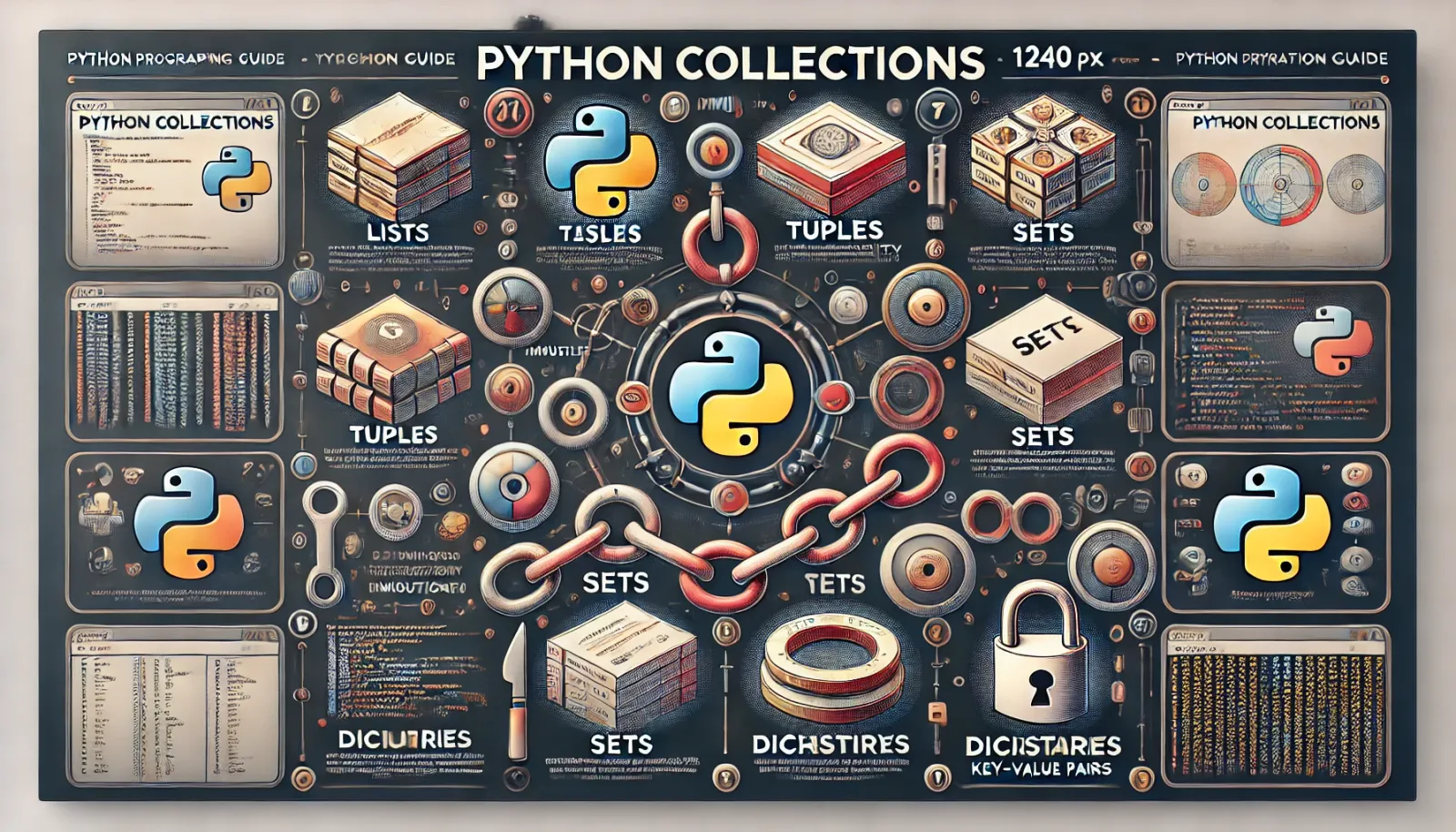
Lists, Tuples, Sets, and Dictionary Overview
Lists: A list is an ordered and mutable collection used to store a series of items. Lists are created by placing elements inside square brackets []
, separated by commas.
fruits = ["apple", "banana", "cherry"]
fruits.append("orange") # Adds 'orange' to the end of the list
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
In the example above, fruits
is a list that initially contains three elements. The append()
method is used to add another element, "orange", to the end of the list.
Tuples: A tuple is an ordered and immutable collection. Tuples are faster than lists and are created by placing elements inside parentheses ()
, separated by commas.
dimensions = (20, 50, 10)
# dimensions[0] = 30 # Uncommenting this line would cause an error as tuples are immutable
print(dimensions[0]) # Access the first element, output: 20
This example creates a tuple dimensions
with three elements. Tuples are immutable, so attempting to change an element throws an error.
Sets: A set is an unordered collection of unique elements. Sets are mutable and are useful for performing mathematical operations like unions, intersections, and set differences.
basket = {"apple", "orange", "apple", "pear", "orange", "banana"}
print(basket) # Output will show unique elements: {'orange', 'banana', 'pear', 'apple'}
basket
is a set that removes duplicate entries automatically. The printed output contains only unique fruits.
Dictionaries: A dictionary is an unordered collection of key-value pairs. Access to items is achieved by keys, which must be unique.
phonebook = {"John": 1234, "Jane": 5678, "Alice": 3456}
phonebook["Dave"] = 9012 # Adds a new entry to the dictionary
print(phonebook["John"]) # Retrieves John's number, output: 1234
In phonebook
, data is stored as pairs of keys (John
, Jane
, Alice
) and values (1234
, 5678
, 3456
). A new key-value pair for "Dave" is added without affecting existing entries.
When to Use Each Collection Type
- Lists: Use lists when you need a collection that can change over time, and you need to preserve the order of your elements. They allow you to store ordered items, making them suitable for tasks where you need to perform iterations, modifications, and have quick access to elements by index.
- Tuples: Since tuples are immutable, they're perfect for storing data that shouldn't change over time. They can be used as keys in dictionaries or as elements of sets, where immutability is necessary.
- Sets: Ideal for membership testing, removing duplicates from a sequence, and performing common math operations like unions, intersections. Use sets when you need to handle unique items and mathematical set operations are required.
- Dictionaries: Dictionaries are optimal when you need a logical association between key:value pairs. When you need fast lookup for data based on custom keys, dictionaries are the preferred choice. They are particularly useful when you're storing and retrieving data via a unique identifier, like names in a phonebook.
Each collection type has its strengths and appropriate use case scenarios. Choosing the right type depends on the requirements of the application and the specific operations you need to perform on the data. For a deeper dive into Python collections, explore these resources:
- Python Collections Module - Provides alternatives and enhancements to the built-in collection types.
- Python Data Structures Tutorial - Offers comprehensive tutorials on each type of collection in Python.