📊 Section 1.4: Data Types in Python
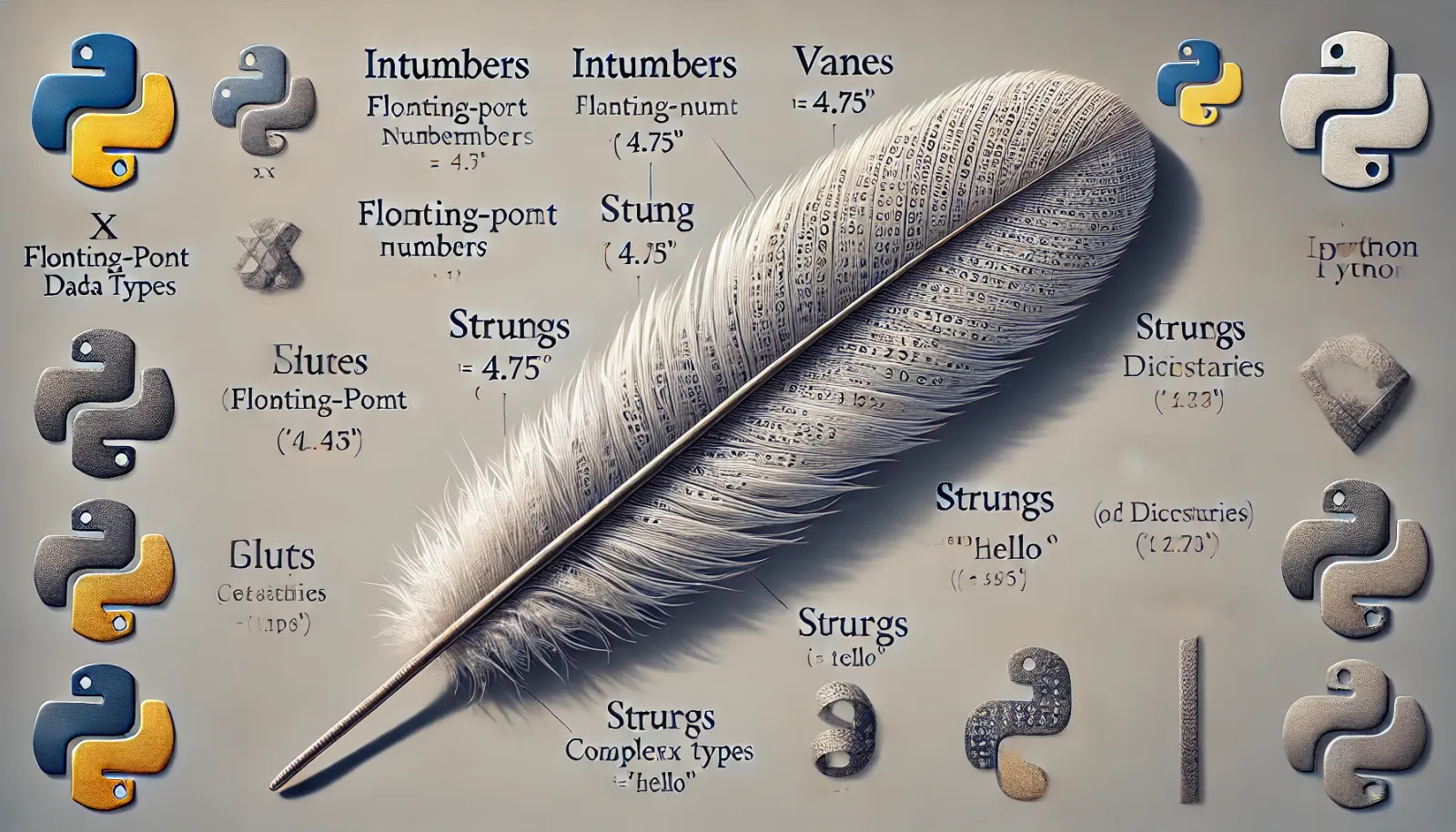
Primitive Data Types: Numbers, Strings, and Booleans
- Numbers: Python supports both integers and floating-point numbers. There’s no need to declare a type; Python automatically handles the type based on the value you assign.
x = 3 # An integer assignment
y = 4.75 # A floating-point number assignment
z = x * y # Multiplication results in a floating-point number
print(z) # This will print 14.25
In this example, x
is an integer, y
is a float, and z
is the result of their multiplication, which Python automatically treats as a float.
- Strings: A string in Python is a sequence of characters. Strings are immutable, meaning that once they are created, the contents cannot be changed.
greeting = "Hello" # A string
name = 'World' # Another string
message = greeting + " " + name + "!" # String concatenation
print(message) # This will print "Hello World!"
Here, greeting
and name
are string variables, and message
combines these with additional text, showcasing string concatenation.
- Booleans: The Boolean type in Python handles two values:
True
andFalse
. It is the result of comparison operations or logical operations.
isActive = True
isRegistered = False
print(isActive and isRegistered) # Logical AND operation, prints False
In this snippet, isActive
is True
and isRegistered
is False
. The and
operation results in False
because both operands are not true.
Complex Data Types: Lists, Tuples, Dictionaries
- Lists: A list in Python is an ordered sequence of items. It is one of the most versatile data types in Python because it can hold items of mixed data types.
numbers = [1, 2, 3, 4, 5] # A list of integers
numbers.append(6) # Adding an element to the end of the list
print(numbers) # Prints [1, 2, 3, 4, 5, 6]
The list numbers
initially contains five elements. The append()
method adds a new element to the end of the list.
- Tuples: Tuples are similar to lists, but they are immutable. Once a tuple is created, you cannot alter its contents.
dimensions = (800, 600) # A tuple
print(dimensions[0]) # Accessing tuple elements, prints 800
# dimensions[0] = 900 # This would result in an error
dimensions
is a tuple that represents screen dimensions. Trying to change an element of a tuple would result in a TypeError because tuples are immutable.
- Dictionaries: A dictionary in Python is an unordered collection of key-value pairs. It allows you to store and retrieve data with keys.
person = {"name": "John", "age": 30, "city": "New York"}
person["email"] = "john@example.com" # Adding a new key-value pair
print(person["name"]) # Accessing value by key, prints "John"
In this dictionary person
, data is stored in key-value pairs. New entries can be added, and existing values can be retrieved using keys.
Understanding these data types is crucial as they form the building blocks for data manipulation and structuring logic in Python programming. For further examples and a deeper exploration, consider checking out the following:
- Python Data Types Documentation - Official Python documentation on standard types.
- Geeks for Geeks Python Programming Examples - Practical examples and tutorials on Python data types.