📦Section 1.10: Creating and Importing Modules
A Python module is simply a Python file containing definitions and statements. Creating your own module can help in organizing code, reusing functionality across multiple scripts, and maintaining a clean codebase.
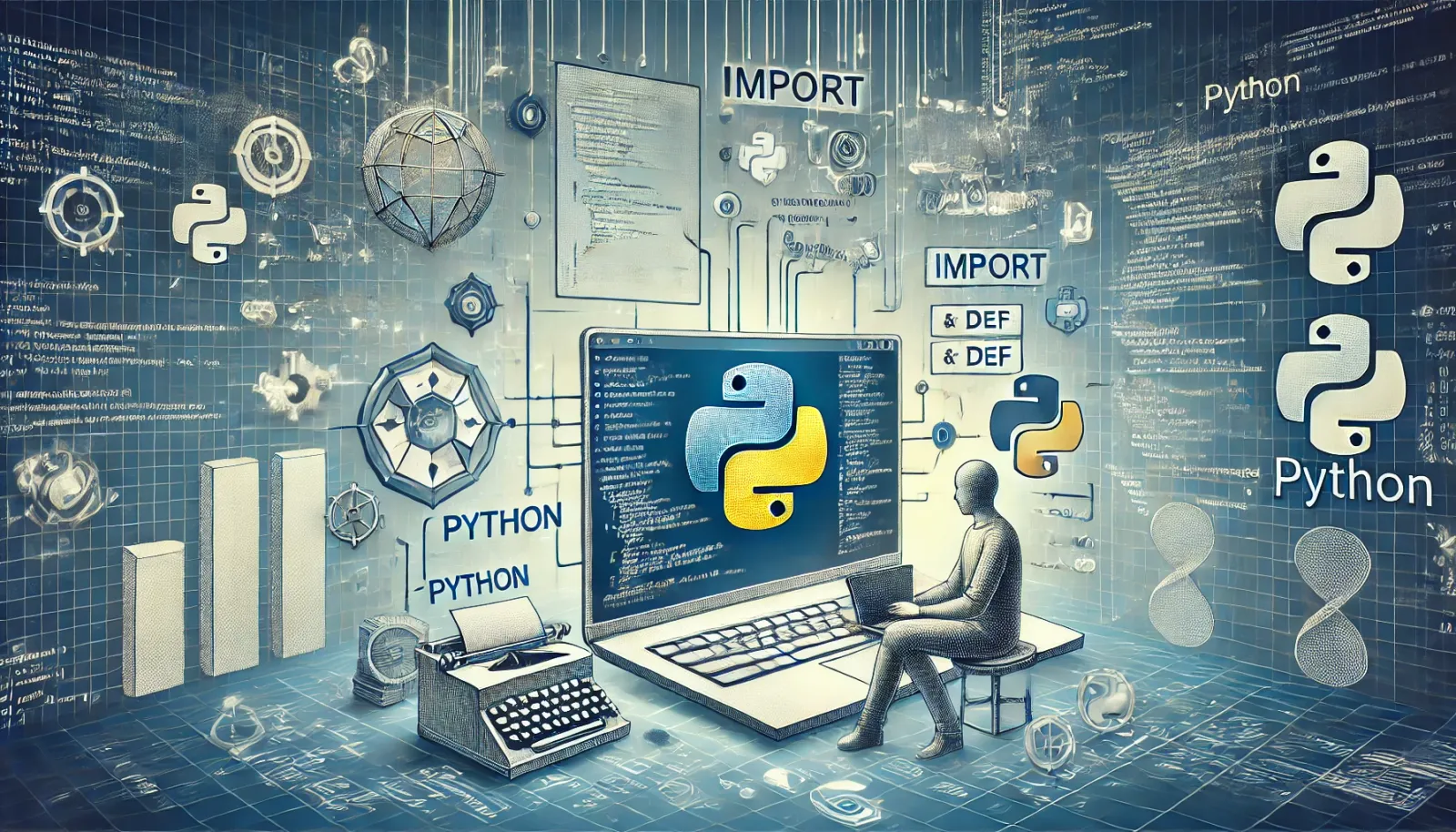
How to Write Your Own Python Module
A Python module is simply a Python file containing definitions and statements. Creating your own module can help in organizing code, reusing functionality across multiple scripts, and maintaining a clean codebase.
Step 1: Writing a Module
To write a Python module, you just need to create a .py
file and define functions, variables, or classes within it.
Example: Creating a simple module named mymodule.py
# mymodule.py
def greet(name):
"""Greet someone by their name."""
return f"Hello, {name}!"
def add_numbers(x, y):
"""Add two numbers."""
return x + y
def greet(name)
: Defines a function that takes a name as input and returns a greeting string.def add_numbers(x, y)
: Defines a function to add two numbers and return the result.
Step 2: Documenting the Module
It is a good practice to include documentation strings (docstrings) for each function to describe what it does.
Importing and Using Modules
Once you have created a module, you can use it in other Python scripts by importing it. This can be done with the import
statement.
Example: Using the mymodule.py
in another script
# Import the entire module
import mymodule
# Using functions from mymodule
result = mymodule.add_numbers(5, 7)
print(result) # Outputs: 12
greeting = mymodule.greet("Alice")
print(greeting) # Outputs: Hello, Alice!
import mymodule
: This line imports the module you created. Python looks for themymodule.py
file in the same directory or in one of the directories listed insys.path
.mymodule.add_numbers(5, 7)
: Calls theadd_numbers
function from the imported module.mymodule.greet("Alice")
: Calls thegreet
function from the imported module.
Example: Importing specific functions from a module
# Import specific functions
from mymodule import greet, add_numbers
# Directly use imported functions
print(greet("Bob")) # Outputs: Hello, Bob!
print(add_numbers(10, 15)) # Outputs: 25
from mymodule import greet, add_numbers
: This line imports specific functions frommymodule
directly. This allows you to use these functions without prefixing them with the module name.
Example: Importing with Aliases
# Importing module with an alias
import mymodule as mm
# Using the alias to call functions
print(mm.greet("Carol")) # Outputs: Hello, Carol!
import mymodule as mm
: Importsmymodule
with an aliasmm
. This can be useful for shortening module names or avoiding naming conflicts.
Best Practices and Tips
- Keep Your Modules Focused: Each module should provide a well-defined and cohesive set of functionalities. Avoid putting too many unrelated functions or classes in the same module.
- Use Meaningful Names: The name of the module should clearly indicate what it contains. This improves readability and maintainability.
- Document Your Modules: Always document what each function or class does. This can be done through docstrings and comments.
Conclusion
Modules in Python provide a simple yet powerful way of organizing and reusing code. By creating your own modules and importing them into your projects, you can keep your codebase clean, manageable, and scalable.