Programming
Programming - Critical for implementing data manipulation, statistical analysis, and algorithm development. Common languages include Python, R, and SQL.
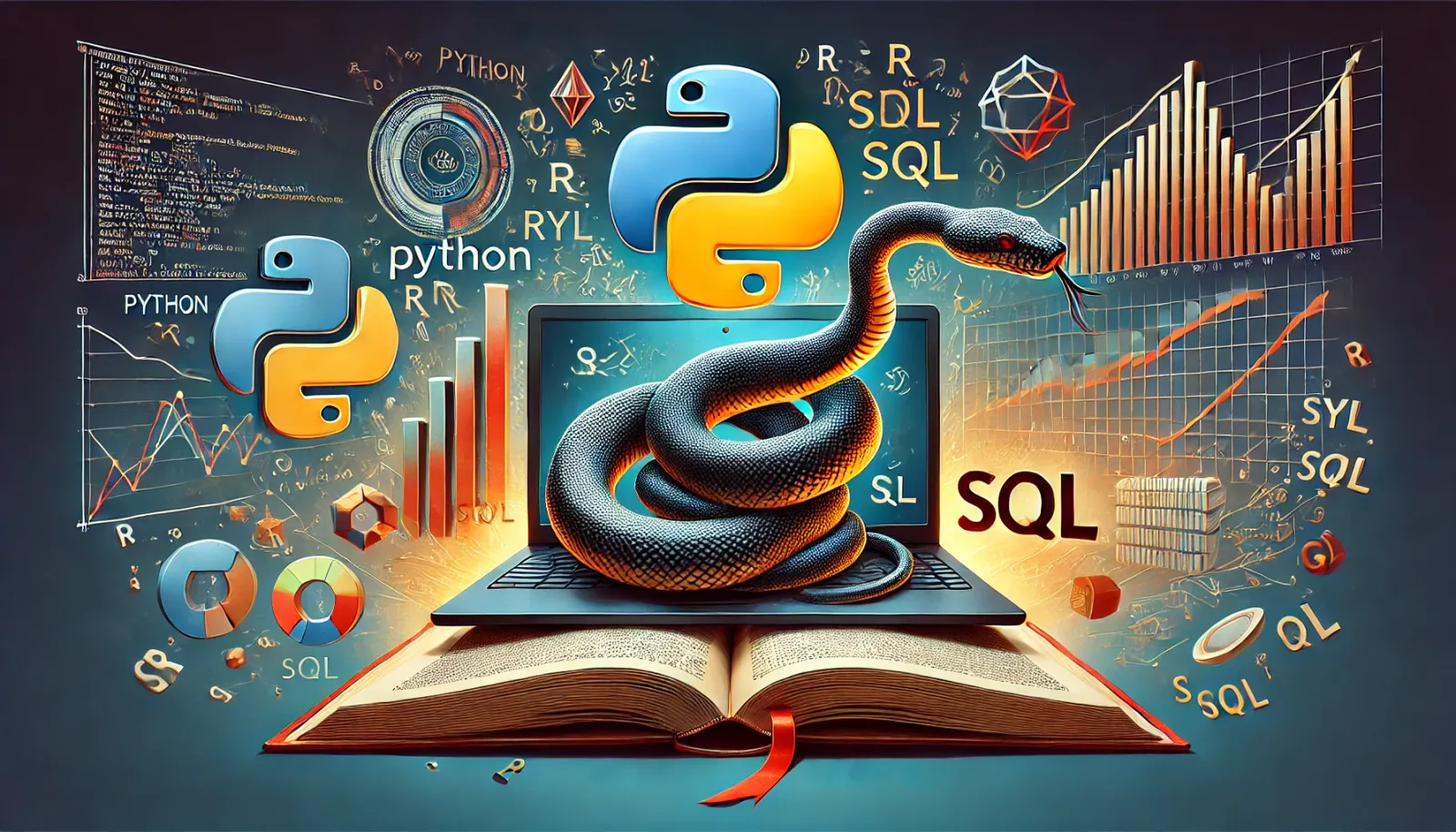
Programming is essential for data scientists, providing the tools to implement data manipulation, statistical analysis, and algorithm development. Proficiency in programming languages like Python, R, and SQL enables data scientists to efficiently work with data, automate tasks, and develop complex models.
1. Introduction to Programming for Data Science
Programming is a fundamental skill for data science, and mastering key programming languages allows professionals to work efficiently with data at scale. Whether you're handling data manipulation, performing statistical analysis, or developing algorithms, you need a strong command of tools like Python, R, and SQL. In this roadmap, we’ll guide you through these languages, showing you how to apply them in data science contexts.
Each language serves a critical role in data analysis:
- Python: A general-purpose language used for scripting, machine learning, and data analysis.
- R: A statistical computing language, excellent for data visualization and statistical modeling.
- SQL: A domain-specific language for managing and querying databases.
2. Python: Foundations and Data Manipulation
Python has become the go-to language for data science because of its flexibility and extensive library ecosystem. Python allows you to manipulate data quickly, making it the most used language for machine learning and data-driven tasks.
- Variables and Data Types: Python supports integers, floats, strings, lists, tuples, and dictionaries—each of which is important for data handling.
- Control Flow: Python's loops (for, while) and conditionals (if, else) allow you to automate repetitive tasks.
- Functions and Modules: You can organize reusable code into functions and separate them into modules, streamlining complex processes.
After learning Python’s basic syntax, the next logical step is using libraries for data manipulation. Python’s rich ecosystem includes libraries such as:
- pandas: The pandas library provides powerful tools to structure and manipulate tabular data through DataFrames.
- NumPy: NumPy offers high-performance array processing, enabling you to perform mathematical computations quickly.
Here’s a basic example of data manipulation using pandas:
import pandas as pd
df = pd.read_csv("data.csv")
filtered_data = df[df['column'] > 100]
3. R: Statistical Computing and Visualization
R is specially designed for statistical analysis and data visualization. For statisticians and data scientists working with large datasets, R is indispensable. It has a strong community and a wealth of packages that simplify complex statistical operations.
- Data Structures in R: R’s core structures include vectors, lists, and data frames. Understanding these is critical to working efficiently with datasets in R.
- Statistical Modeling: R simplifies statistical modeling, making it easy to apply linear and logistic regression, hypothesis testing, and much more.
- ggplot2 for Visualization: ggplot2, a popular R package, allows for creating visually compelling graphs with minimal effort.
R’s power lies in its ability to combine advanced statistical computing with sophisticated visualizations. Here’s how you can create a plot using ggplot2:
library(ggplot2)
ggplot(data, aes(x=var1, y=var2)) + geom_point()
4. SQL: Managing and Querying Databases
SQL (Structured Query Language) is the foundation of database management. Whether you’re dealing with small or massive datasets, SQL allows you to retrieve, update, and analyze data stored in relational databases efficiently.
- Basic SQL Queries: Start with the fundamental SQL query—the
SELECT
statement, which retrieves data from tables. From there, you’ll use clauses likeWHERE
,GROUP BY
, andORDER BY
to refine your queries. - Joins: SQL's ability to perform joins (INNER, LEFT, RIGHT) allows data from multiple tables to be combined for relational analysis.
- Subqueries: Subqueries allow for more complex querying inside larger statements, making SQL even more powerful for data manipulation.
Here's an example of a basic SQL query:
SELECT name, salary FROM employees WHERE salary > 50000;
5. Advanced Python: Machine Learning and Data Science
Python’s versatility shines in machine learning. With powerful libraries such as scikit-learn, TensorFlow, and Keras, Python enables the development of sophisticated machine learning models.
- Supervised Learning: Algorithms like linear regression and decision trees are used for predictive tasks where the outcome is known (e.g., house price prediction).
- Unsupervised Learning: Clustering algorithms (like K-means) help uncover hidden patterns in unlabeled datasets.
- Neural Networks and Deep Learning: Libraries like TensorFlow and Keras enable the development of deep learning models for tasks such as image classification.
Here's a simple machine learning model using scikit-learn:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
6. Final Steps: Mastery of Programming in Data Science
Now that you’ve covered the fundamentals of programming in Python, R, and SQL, it's time to apply your knowledge to real-world projects. Mastery involves continuous learning, collaboration, and problem-solving.
- Work on open-source projects on platforms like GitHub to gain practical experience.
- Participate in data science competitions on websites like Kaggle.
- Keep expanding your knowledge by reading papers, blogs, and documentation.
- Collaborate with professionals in various industries to apply programming to finance, healthcare, and marketing.