Day5: Feature Engineering
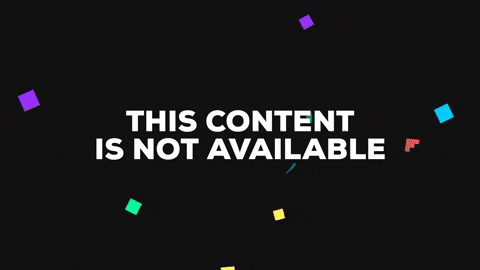
๐ Table of Contents
- ๐ Welcome to Day 5
- ๐ง What is Feature Engineering?
- ๐ก Why is Feature Engineering Important?
- ๐ Key Feature Engineering Techniques
- Feature Creation
- Feature Selection
- Dimensionality Reduction
- Binning and Discretization
- Interaction Features
- Domain-Specific Transformations
- ๐๏ธ Practical Techniques and Code Examples
- Creating New Features
- Feature Selection with SelectKBest
- PCA for Dimensionality Reduction
- Polynomial Features for Interactions
- ๐ Integrating Feature Engineering with EDA and Preprocessing
- ๐ป Practical Examples and Use Cases
- ๐ Resources
- ๐ก Tips and Tricks
1. ๐ Welcome to Day 5
Welcome to Day 5 of your 90-day machine learning journey! Having tackled data preprocessing, we now move on to Feature Engineeringโthe process of extracting, transforming, and selecting the most meaningful representations of data.
Feature engineering is where domain knowledge meets technical skill. By carefully crafting features, you can dramatically enhance a modelโs predictive power and interpretability. Instead of relying solely on raw data, well-engineered features provide clearer signals for the model to learn from, leading to better performance and deeper insights.
2. ๐ง What is Feature Engineering?
Feature Engineering involves creating new variables or transforming existing ones to better represent the underlying patterns in the data. Rather than using raw features as-is, you manipulate them to highlight relationships that might be hidden.
Examples:
- Converting timestamps into โday of weekโ or โhour of dayโ features.
- Aggregating transaction records to create โtotal monthly spendโ for each customer.
- Extracting text length or keyword counts from documents.
Related Image (Feature Engineering Concept):

(Image Source: Intelliarts)
3. ๐ก Why is Feature Engineering Important?
Models are only as good as the data they learn from. Even the most advanced algorithm can falter if the features lack meaningful patterns. Feature engineering:
- Boosts Model Accuracy: Well-crafted features can make patterns more obvious to the model.
- Reduces Complexity: Feature selection and dimensionality reduction strip away noise, focusing the model on the most informative signals.
- Leverages Domain Knowledge: Incorporating expert insights can lead to novel features that pure algorithms might miss.
- Improves Interpretability: Transparent features help stakeholders understand why a model makes certain predictions.
4. ๐ Key Feature Engineering Techniques
๐ Feature Creation
Transform raw data into more informative forms. For example, from a date of birth you can create age; from latitude and longitude, you can derive distances or regions.
Related Image (Feature Creation):

(Image Source: KDnuggets)
๐ Feature Selection
Remove irrelevant or redundant features to reduce noise. Fewer, better-chosen features can speed up training and improve generalization.
Related Image (Feature Selection):

(Image Source: Medium)
๐ Dimensionality Reduction
Techniques like PCA help reduce thousands of features into a few principal components without losing too much information.
Related Image (Dimensionality Reduction with PCA):

(Image Source: Wikimedia)
๐ Binning and Discretization
Group continuous values into bins or categories. For example, grouping ages into ranges (0-18, 19-35, 36-50, 51+).
Related Image (Binning Illustration):

(Image Source: Statistics How To)
๐ Interaction Features
Combine two or more features to reveal relationships. For example, multiply โpriceโ and โquantityโ to get โtotal revenueโ.
Related Image (Feature Interactions):

(Image Source: Analytics Vidhya)
๐ Domain-Specific Transformations
Use field knowledge to apply transformations. In finance, you might log-transform transaction amounts; in image analysis, you might extract edges or colors.
Related Image (Domain-Specific Features):

(Image Source: FeatureEngineeringBook)
5. ๐๏ธ Practical Techniques and Code Examples
๐ Creating New Features
import pandas as pd
df = pd.DataFrame({
'full_name': ['John Doe', 'Jane Smith', 'Alice Johnson'],
'birth_year': [1990, 1985, 1975],
'purchase_amt': [120.5, 80.0, 230.7]
})
# Create age feature based on current year (assume 2024)
df['age'] = 2024 - df['birth_year']
# Extract first and last names
df[['first_name','last_name']] = df['full_name'].str.split(' ', expand=True)
# Create a log-transformed purchase amount feature
import numpy as np
df['log_purchase_amt'] = np.log(df['purchase_amt']+1)
print(df)
๐ Feature Selection with SelectKBest
from sklearn.datasets import load_iris
from sklearn.feature_selection import SelectKBest, f_classif
iris = load_iris()
X = iris.data
y = iris.target
selector = SelectKBest(score_func=f_classif, k=2)
X_selected = selector.fit_transform(X, y)
print("Selected features shape:", X_selected.shape)
๐ PCA for Dimensionality Reduction
from sklearn.decomposition import PCA
pca = PCA(n_components=2)
X_pca = pca.fit_transform(X)
print("Reduced shape:", X_pca.shape)
๐ Polynomial Features for Interactions
from sklearn.preprocessing import PolynomialFeatures
poly = PolynomialFeatures(degree=2, interaction_only=True, include_bias=False)
X_poly = poly.fit_transform(X[:, :2]) # Use only first 2 features for demonstration
print("Shape with interaction features:", X_poly.shape)
6. ๐ Integrating Feature Engineering with EDA and Preprocessing
Feature engineering doesnโt happen in a vacuum. Before creating or selecting features, EDA and preprocessing guide your decisions:
- EDA: Identify patterns, relationships, and distributions that suggest potential features.
- Preprocessing: Ensure that your raw data is clean and consistent before engineering features on top of it.
For example:
- After EDA, you notice a seasonality pattern in sales data โ create a โmonthโ or โday_of_weekโ feature.
- After preprocessing, you have no missing values, so you can confidently derive new ratios or sums.
Related Image (Data Pipeline):

(Image Source: ml4devs)
7. ๐ป Practical Examples and Use Cases
-
Fraud Detection:
Create features like โaverage transaction amount in the last weekโ or โtime since last transaction.โ Combine these with customer demographics to enhance fraud detection models. -
Marketing Analytics:
For customer segmentation, derive โcustomer lifetime value,โ โchurn probability,โ or โaverage order frequency.โ Use domain knowledge to engineer features that reflect customer behavior. -
Text Classification:
From raw text, extract features like word counts, TF-IDF scores, sentiment polarity, or named entity counts. These engineered features often outperform raw text inputs.
Related Image (Real-World Use Cases):

(Image Source: Analytics Vidhya)
8. ๐ Resources
- Feature Engineering & Selection Book: Comprehensive resource on engineering and selecting features.
- Kaggle Kernels: Explore community solutions to see how top performers engineer features.
- Scikit-Learnโs Feature Engineering Guides: Official docs on transformations.
- [Blogs & Tutorials]:
- Medium, Towards Data Science, and Analytics Vidhya often have step-by-step articles on feature engineering techniques.
- [Courses]:
- Coursera (Feature Engineering in Big Data Analytics)
- Udemy (Feature Engineering for Machine Learning)
9. ๐ก Tips and Tricks
- Think Creatively: Consider time-based, aggregated, and domain-specific features that capture subtle patterns.
- Less Can Be More: Not all features help. After engineering new features, test performance and drop the less useful ones.
- Automate with Pipelines: Use scikit-learn pipelines to apply transformations systematically.
- Iterate: Feature engineering is an iterative process. As you learn more about your data, refine and improve your features.
Related Image (Continuous Improvement):

(Image Source: MyGreatLearning)
Conclusion: Feature engineering is a powerful tool in a data scientistโs arsenal. By crafting better features, you give your models a clear roadmap to understanding complex data. While algorithms are crucial, itโs often the quality of your features that sets great models apart from good ones. Embrace experimentation, domain insight, and creativity as you engineer features that unlock new levels of model performance!
Next steps? Putting feature engineering into practice on your datasets!