The Arena of Algorithmic Challenge and Innovation
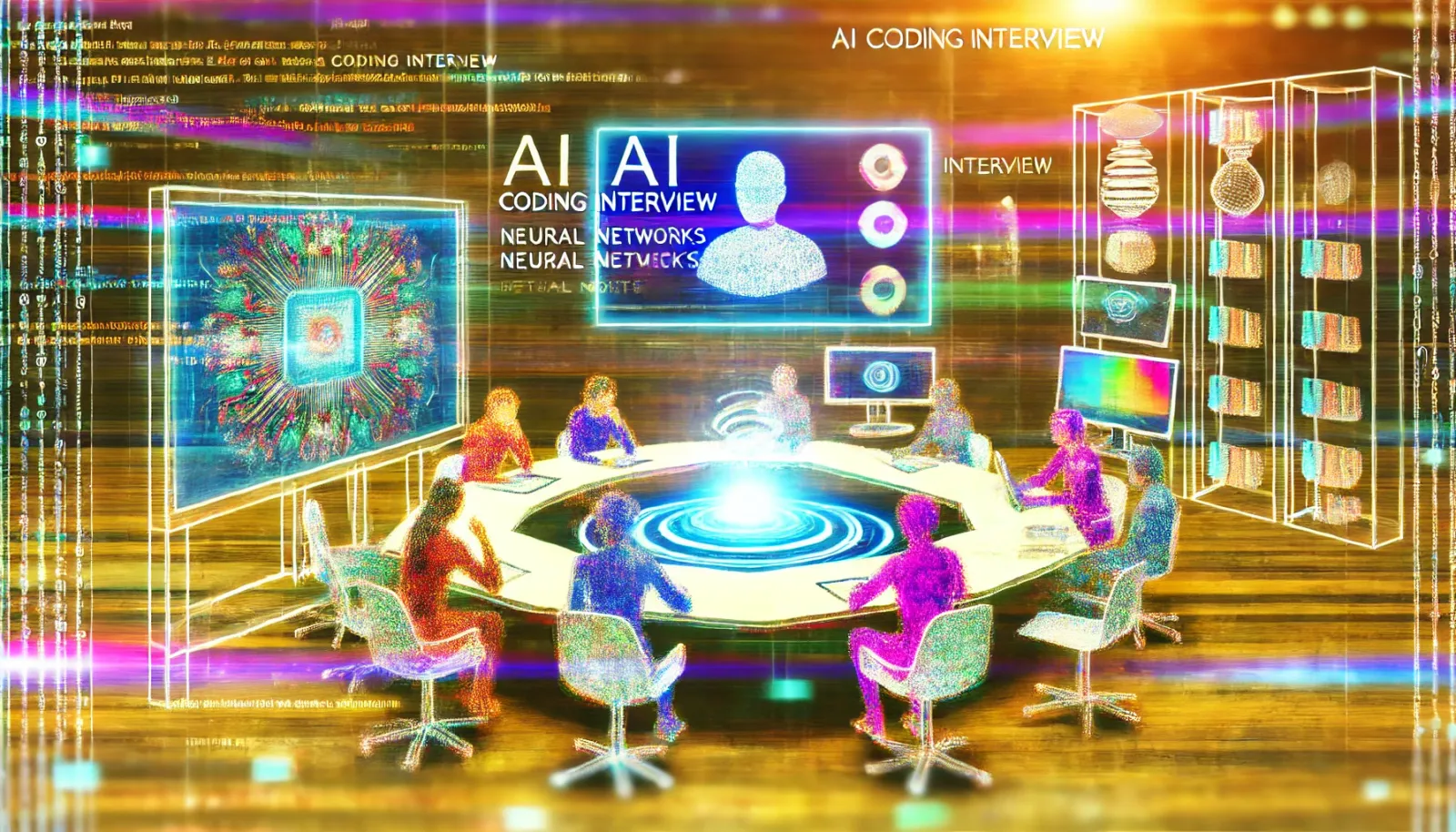
Esteemed AI Enthusiast,
You are about to embark on an exhilarating journey where coding meets creativity, and challenges serve as gateways to groundbreaking innovations in Artificial Intelligence. This interview is not merely an assessment of your technical abilities; it is a celebration of your potential to shape the future of AI. As you prepare to showcase your talents, envision yourself as a key player in an epic saga of innovation that will redefine industries, enhance daily life, and unlock mysteries through the power of AI.
Embark on Your Odyssey in AI Development
This coding interview is crafted to probe the depths of your technical expertise and uncover your innovative approach to solving complex problems using Artificial Intelligence. It’s an opportunity to demonstrate how your skills align with the grand challenges of AI, from machine learning to neural networks, from natural language processing to robotics, and beyond.
Engage with Pioneers of AI Revolution
Step into an environment where each problem you solve and each line of code you write contributes to the monumental task of advancing AI technology. You will engage with some of the brightest minds in the field—visionaries who are pushing the boundaries of what AI can achieve. This interview is your gateway to join a league of extraordinary thinkers who are not just part of the AI revolution but are leading it.
Transform Challenges into Opportunities
Today, you will tackle challenges that mirror the complexities and nuances of real-world AI applications. Each task is designed to not only test your coding prowess but also to stimulate your creative thinking and problem-solving skills in areas such as computational intelligence, predictive analytics, and automated reasoning.
A Platform to Propel Your Passions
We are excited to witness how you leverage AI to turn theoretical concepts into practical solutions. This interview is your platform to shine, a stage to share how your unique insights and innovative approaches can contribute to the evolving landscape of Artificial Intelligence.
Sample Coding Interview Questions with Code-Related Answers
Question 1: Write a Python function to identify and replace all integers in a given list that are less than a specified number with the average of the list. Use AI to predict what the threshold should be.
def replace_with_average(numbers, threshold=None):
import numpy as np
from sklearn.ensemble import RandomForestRegressor
if threshold is None:
# Using a RandomForestRegressor to predict the threshold based on a synthetic feature
X = np.array(numbers).reshape(-1, 1)
y = np.array(numbers)
reg = RandomForestRegressor(n_estimators=10)
reg.fit(X, y)
threshold = reg.predict(np.array([[np.mean(numbers)]]))[0]
average = np.mean(numbers)
return [average if num < threshold else num for num in numbers]
# Example usage:
numbers = [10, 20, 15, 5, 30, 25]
modified_list = replace_with_average(numbers)
print("Modified List:", modified_list)
Question 2: Develop a Python script that uses NLP to summarize text data and then apply sentiment analysis to determine the overall tone of the summary.
from transformers import pipeline
def summarize_and_analyze(text):
# Load summarization and sentiment analysis pipelines
summarizer = pipeline("summarization")
sentiment_analyzer = pipeline("sentiment-analysis")
# Generate summary
summary = summarizer(text, max_length=50, min_length=25, do_sample=False)[0]['summary_text']
# Analyze sentiment
sentiment = sentiment_analyzer(summary)[0]
return summary, sentiment
# Example usage:
text = "Artificial Intelligence has been one of the most revolutionary aspects of technology. It's transforming industries, enhancing capabilities, and providing insights into previously unsolvable problems."
summary, sentiment = summarize_and_analyze(text)
print("Summary:", summary)
print("Sentiment:", sentiment)
A New Era of AI Awaits Your Contribution
As you step into this interview, remember that you are not just solving coding problems—you are laying the groundwork for future AI solutions that could revolutionize the way we live and work. We eagerly anticipate your innovative ideas and are excited to explore how your vision and skills can contribute to the broader AI initiatives at our company.
Welcome, and let’s together push the boundaries of what AI can achieve.
Prepare to inspire and be inspired. The journey to redefine the future of AI starts now. We look forward to discovering your potential and exploring the possibilities together. See you at the forefront of AI innovation!