Explain object-oriented programming concepts
Object-oriented programming (OOP) organizes code into reusable objects using classes, focusing on encapsulation, inheritance, polymorphism, and abstraction.
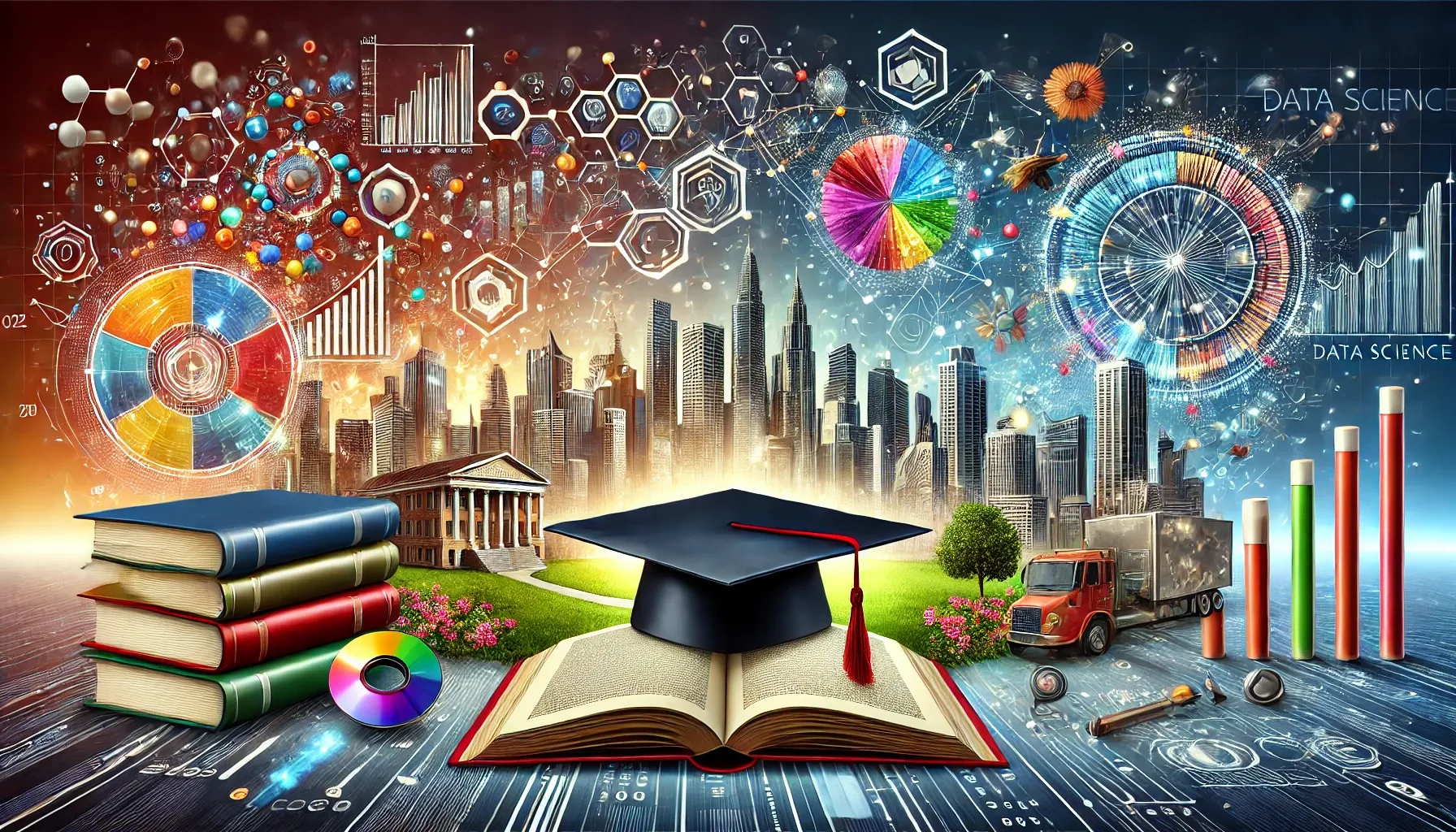
Object-oriented programming (OOP) is a programming paradigm centered around the concept of "objects," which are instances of classes. OOP enables more modular, reusable, and maintainable code by organizing software design around these objects. Here are the key concepts of OOP:
1. Classes and Objects
- Class: A class is a blueprint or template for creating objects. It defines a set of attributes (data) and methods (functions) that the objects created from the class will have.
- Object: An object is an instance of a class. When a class is defined, no memory is allocated until an object of that class is created. Each object can have unique values for its attributes.
Example:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def start_engine(self):
print("Engine started")
my_car = Car("Toyota", "Camry", 2020)
my_car.start_engine() # Output: Engine started
2. Encapsulation
- Encapsulation is the concept of bundling the data (attributes) and the methods that operate on the data into a single unit, i.e., the class. It also involves restricting access to certain components of the object, which is typically done using access modifiers like
private
,protected
, andpublic
. - This ensures that the internal representation of the object is hidden from the outside, providing a way to control how the data is accessed or modified.
Example:
class BankAccount:
def __init__(self, balance):
self.__balance = balance # private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
def get_balance(self):
return self.__balance
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
3. Inheritance
- Inheritance allows a new class to inherit attributes and methods from an existing class. The new class, known as the subclass (or derived class), can also have additional attributes and methods or override existing ones.
- This promotes code reuse and establishes a relationship between classes.
Example:
class Vehicle:
def __init__(self, make, model):
self.make = make
self.model = model
def start(self):
print("Starting the vehicle")
class Car(Vehicle):
def __init__(self, make, model, doors):
super().__init__(make, model)
self.doors = doors
def start(self):
print("Starting the car")
my_car = Car("Honda", "Civic", 4)
my_car.start() # Output: Starting the car
4. Polymorphism
- Polymorphism means "many forms" and refers to the ability of different classes to be treated as instances of the same class through inheritance. It allows methods to do different things based on the object it is acting upon, even if they share the same name.
- Polymorphism is achieved through method overriding and method overloading (in some languages).
Example:
class Bird:
def make_sound(self):
print("Some generic bird sound")
class Parrot(Bird):
def make_sound(self):
print("Squawk")
class Crow(Bird):
def make_sound(self):
print("Caw")
def sound_test(bird):
bird.make_sound()
parrot = Parrot()
crow = Crow()
sound_test(parrot) # Output: Squawk
sound_test(crow) # Output: Caw
5. Abstraction
- Abstraction is the concept of hiding the complex implementation details and showing only the essential features of an object. It helps reduce programming complexity by focusing on the interactions with the object rather than the internal workings.
- Abstract classes and interfaces are commonly used to implement abstraction in many OOP languages.
Example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
rect = Rectangle(5, 10)
print(rect.area()) # Output: 50
Summary
- Classes are blueprints for objects.
- Objects are instances of classes.
- Encapsulation protects object integrity by restricting access to its internals.
- Inheritance allows new classes to extend existing ones.
- Polymorphism enables methods to process objects differently based on their class.
- Abstraction hides implementation details, focusing on object interactions.
These principles make OOP a powerful paradigm for designing software that is modular, maintainable, and scalable.