Dictionaries in Python: Key-Value Pairs, Accessing & Modifying Data, Use Cases in Data Manipulation
โ Mejbah Ahammad
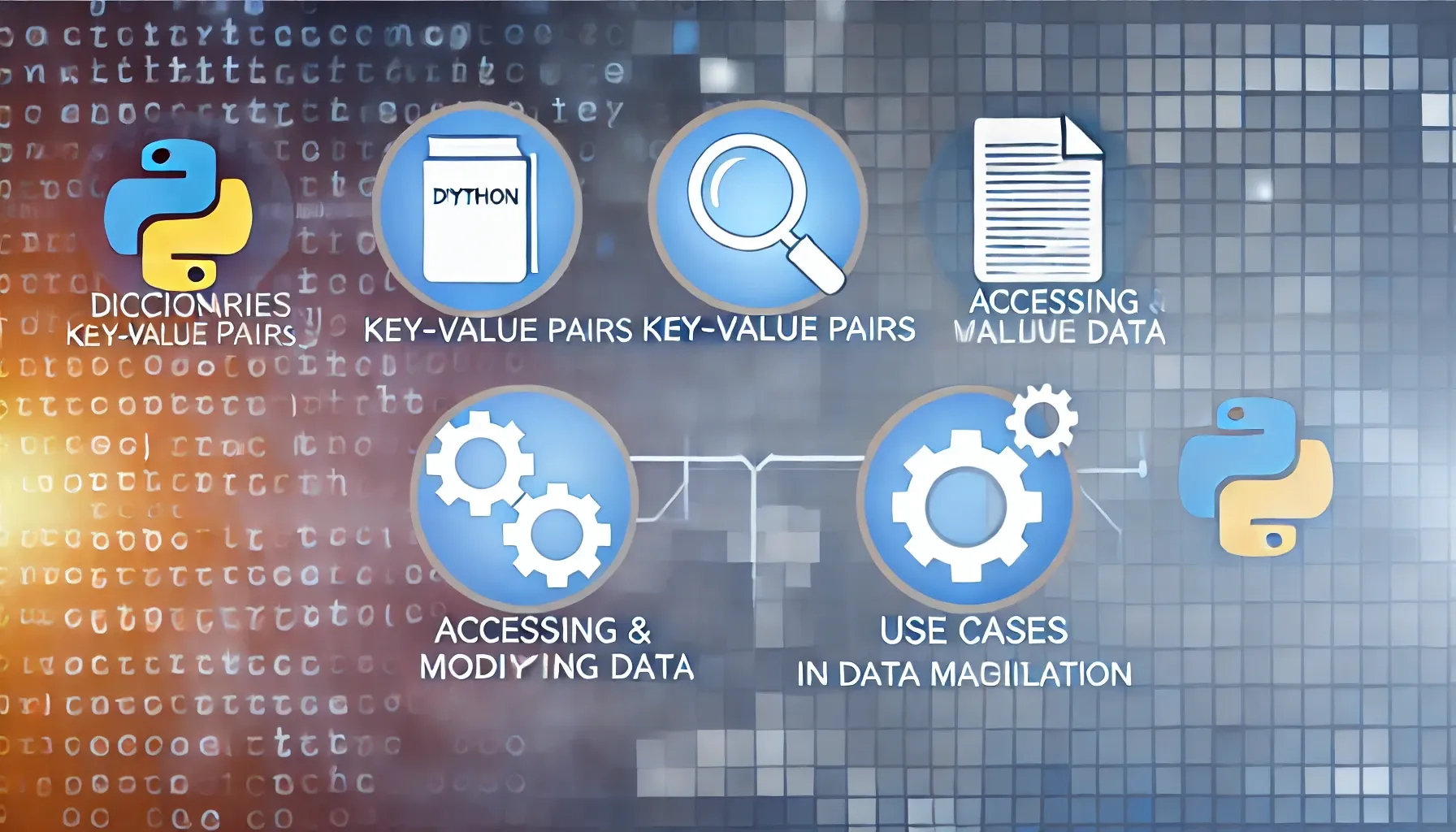
๐ Dictionaries in Python: Key-Value Pairs, Accessing & Modifying Data, Use Cases in Data Manipulation
Dictionaries are one of the most versatile and widely used data structures in Python. They store data in the form of key-value pairs, providing an efficient way to retrieve, modify, and manipulate data. In this guide, weโll explore ๐ก how dictionaries work, โ how to access and modify them, and โ various use cases where dictionaries excel in data manipulation tasks. Letโs dive in! ๐
๐ณ Creating a Dictionary
A dictionary is created using curly braces {}
, where each element consists of a key and its associated value separated by a colon. The key must be unique, and it can be any immutable data type such as strings, numbers, or tuples. The value, on the other hand, can be of any data type, including lists, other dictionaries, and more.
# Creating a dictionary person = { "name": "Alice", "age": 30, "city": "New York", "is_student": False } print(person)
In the example above, we created a dictionary called person
that holds key-value pairs for a personโs name, age, city, and student status. Each key is unique and is used to access the corresponding value. ๐ณ
๐ Accessing Data in a Dictionary
You can access values in a dictionary by using their corresponding keys. If you try to access a key that doesnโt exist, Python will raise a KeyError
, so itโs often a good idea to use the get()
method, which returns None
if the key is not found.
# Accessing values using keys name = person["name"] age = person.get("age") city = person.get("country", "Unknown") # Provides a default value if key doesn't exist print(f"Name: {name}, Age: {age}, City: {city}")
Here, we accessed the values of "name" and "age" directly. The get()
method allows us to provide a default value in case the key "country" is not found, which avoids raising an error. ๐
๐ฟ Modifying Data in a Dictionary
Dictionaries are mutable, which means you can update, add, or remove key-value pairs after they are created. Letโs look at how to modify data in a dictionary.
โ Updating Values
You can update the value associated with a specific key by assigning a new value to it.
# Updating a value person["age"] = 31 # Update age person["city"] = "San Francisco" # Change city print(person)
โ Adding New Key-Value Pairs
To add a new key-value pair, simply assign a value to a new key that doesnโt yet exist in the dictionary.
# Adding a new key-value pair person["email"] = "alice@example.com" print(person)
โ Removing Key-Value Pairs
You can remove key-value pairs using the del
statement, pop()
method, or popitem()
method, which removes the last key-value pair in Python 3.7+.
# Removing a key-value pair del person["is_student"] # Using del # Using pop to remove and return the value of the key removed_value = person.pop("email") print(f"Updated dictionary: {person}") print(f"Removed value: {removed_value}")
The del
statement is used to remove the is_student
key, while pop()
removes the "email" key and returns its value. ๐ฟ
๐ก Use Cases for Dictionaries
Dictionaries are particularly useful for situations where you need to map unique keys to values and perform quick lookups. Below are some common use cases where dictionaries excel in data manipulation.
โ Storing Configuration Data
Dictionaries are perfect for storing configuration settings, where each setting has a unique key and a value that can be easily accessed and updated if needed.
# Configuration settings stored in a dictionary config = { "theme": "dark", "language": "en", "auto_save": True } # Access a setting theme = config.get("theme") print(f"Theme: {theme}") # Update a setting config["auto_save"] = False print(f"Updated config: {config}")
โ Counting Elements in a Dataset
Dictionaries can be used to count occurrences of items in a list or other iterable. For example, counting the frequency of words in a text.
# Counting word frequency using a dictionary text = "apple banana apple cherry banana apple" word_list = text.split() word_count = {} for word in word_list: if word in word_count: word_count[word] += 1 else: word_count[word] = 1 print(word_count)
In this example, the dictionary word_count
is used to store the frequency of each word in the text. Each word is a key, and the count is the value. โ
โ Storing Data in a Structured Format
Dictionaries can store data in a structured and nested format, such as when representing a dataset where each key is a record ID, and the value is another dictionary of details.
# Storing student data in a nested dictionary students = { 101: {"name": "Alice", "grade": "A", "age": 20}, 102: {"name": "Bob", "grade": "B", "age": 21}, 103: {"name": "Charlie", "grade": "A", "age": 22} } # Accessing a student's data alice_data = students[101] print(f"Alice's Data: {alice_data}") # Modifying a student's grade students[102]["grade"] = "A" print(f"Updated Students: {students}")
This nested dictionary stores data for students where the student ID is the key, and the value is another dictionary holding the student's details. This is a common structure used in databases, configuration files, and more. ๐ก
๐ฑ Dictionary Comprehensions
Python offers a powerful feature called **dictionary comprehensions**, which allows you to construct dictionaries in a concise and readable way using a loop-like syntax.
# Dictionary comprehension example squares = {x: x**2 for x in range(1, 6)} print(f"Squares Dictionary: {squares}")
The example above shows how we can create a dictionary that maps numbers to their squares using a single line of code. This is much more concise than traditional for loops. ๐ฑ
๐ Conclusion
Dictionaries are one of Pythonโs most efficient and flexible data structures for organizing and manipulating data. By mastering key-value pairs, accessing, and modifying data, you can handle complex datasets, configurations, and perform tasks such as counting occurrences or mapping records. With practical features like dictionary comprehensions and nested dictionaries, Python dictionaries are essential tools for any data manipulation tasks. โ